写一个基于hadoop的倒排索引代码
时间: 2023-07-04 17:08:02 浏览: 87
倒排索引是一种经典的信息检索技术,它将每个单词映射到包含该单词的文档列表中。在Hadoop中,我们可以使用MapReduce来构建倒排索引。
下面是一个简单的基于Hadoop的倒排索引代码示例:
1. 首先,我们需要编写一个Mapper类来处理输入数据并输出中间结果。Mapper的任务是将每个单词映射到包含该单词的文档列表中。这里我们假设每个输入文件包含一行文本,每个文本包含多个单词。
```
public static class InvertedIndexMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text word = new Text();
private Text docId = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// 获取输入行
String line = value.toString();
// 获取文档ID
int pos = line.indexOf('\t');
String docIdStr = line.substring(0, pos);
docId.set(docIdStr);
// 获取文本内容
String text = line.substring(pos + 1);
// 将文本内容分割为单词
StringTokenizer tokenizer = new StringTokenizer(text);
while (tokenizer.hasMoreTokens()) {
String token = tokenizer.nextToken();
word.set(token);
context.write(word, docId);
}
}
}
```
2. 接下来,我们需要编写一个Reducer类来将Mapper输出的中间结果合并为最终的倒排索引。Reducer的任务是将每个单词映射到包含该单词的文档列表中。
```
public static class InvertedIndexReducer extends Reducer<Text, Text, Text, Text> {
private Text result = new Text();
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
// 构造文档ID列表
StringBuilder sb = new StringBuilder();
for (Text val : values) {
sb.append(val.toString());
sb.append(",");
}
String docList = sb.toString();
docList = docList.substring(0, docList.length() - 1);
// 输出倒排索引
result.set(docList);
context.write(key, result);
}
}
```
3. 最后,我们需要编写一个Driver类来配置和启动MapReduce作业。
```
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Inverted Index");
job.setJarByClass(InvertedIndex.class);
job.setMapperClass(InvertedIndexMapper.class);
job.setReducerClass(InvertedIndexReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
```
以上代码实现了一个基于Hadoop的倒排索引,可以使用以下命令来运行:
```
hadoop jar inverted-index.jar input output
```
其中,`input`是输入文件的路径,`output`是输出文件的路径。输出文件将包含每个单词映射到包含该单词的文档列表中的倒排索引。
相关推荐
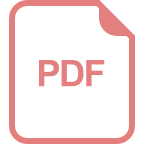
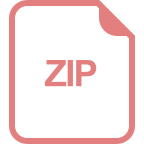
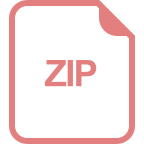














