Hadoop 倒排索引
时间: 2024-02-10 17:08:02 浏览: 35
Hadoop倒排引是一种用于快速查找文档中特定单词的技术。它通过将文档中的每个单词映射到包含该单词的文档列表来实现。在Hadoop中,倒排索引可以通过MapReduce作业来创建。以下是创建Hadoop倒排索引的一些步骤:
1.将文档分成单词并将每个单词映射到文档ID。这可以通过MapReduce作业中的Mapper来完成。在Mapper中,您可以使用FileSplit对象获取当前正在处理的文档的名称或ID。
2.将单词列表按字母顺序排序。这可以通过MapReduce作业中的Reducer来完成。在Reducer中,您可以使用TreeMap来对单词进行排序。
3.将单词列表写入输出文件。这可以通过MapReduce作业中的OutputFormat来完成。在OutputFormat中,您可以使用SequenceFileOutputFormat来将单词列表写入二进制文件中,以便后续的MapReduce作业可以更快地读取它们。
以下是一个简单的Hadoop倒排索引的示例代码:
```java
import java.io.IOException;
import java.util.StringTokenizer;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.input.FileSplit;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class InvertedIndex {
public static class TokenizerMapper
extends Mapper<Object, Text, Text, Text>{
private Text word = new Text();
private Text docId = new Text();
public void map(Object key, Text value, Context context
) throws IOException, InterruptedException {
StringTokenizer itr = new StringTokenizer(value.toString());
while (itr.hasMoreTokens()) {
word.set(itr.nextToken());
docId.set(((FileSplit) context.getInputSplit()).getPath().getName());
context.write(word, docId);
}
}
}
public static class IntSumReducer
extends Reducer<Text,Text,Text,Text> {
private Text result = new Text();
public void reduce(Text key, Iterable<Text> values,
Context context
) throws IOException, InterruptedException {
StringBuilder sb = new StringBuilder();
for (Text val : values) {
sb.append(val.toString());
sb.append(",");
}
result.set(sb.toString());
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "inverted index");
job.setJarByClass(InvertedIndex.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class);
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
相关推荐
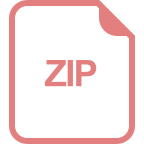
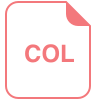
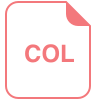
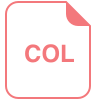
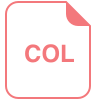
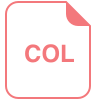








