用java写一个mapreduce数据清洗案例
时间: 2024-05-24 22:13:03 浏览: 182
以下是一个基于Java的MapReduce数据清洗案例:
假设有一个数据集,包含多行记录,每行记录由三个字段组成:name, age和gender。其中,name字段可能会包含特殊字符或空格,age字段可能会包含非数字字符或负数,而gender字段可能会包含空格或大小写不一致的字母。
我们想要清洗这个数据集,将name字段中的特殊字符和空格去除,将age字段中的非数字字符和负数去除,将gender字段中的空格去除并将字母转换为小写。
下面是实现这个数据清洗过程的Java代码:
Mapper类:
public class DataCleanMapper extends Mapper<LongWritable, Text, Text, Text>{
private Text outputKey = new Text();
private Text outputValue = new Text();
@Override
protected void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
String name = fields[0].replaceAll("[^a-zA-Z0-9]", "").trim();
String ageStr = fields[1].replaceAll("[^0-9]", "").trim();
int age = Integer.parseInt(ageStr);
if(age <= 0){
return;
}
String gender = fields[2].replaceAll("\\s+", "").toLowerCase();
outputKey.set(name);
outputValue.set(age + "\t" + gender);
context.write(outputKey, outputValue);
}
}
Reducer类:
public class DataCleanReducer extends Reducer<Text, Text, Text, Text>{
private Text outputValue = new Text();
@Override
protected void reduce(Text key, Iterable<Text> values, Context context)
throws IOException, InterruptedException {
for(Text value : values){
outputValue.set(value);
context.write(key, outputValue);
break;
}
}
}
Driver类:
public class DataCleanDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "data clean");
job.setJarByClass(DataCleanDriver.class);
job.setMapperClass(DataCleanMapper.class);
job.setReducerClass(DataCleanReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
在这个案例中,Mapper类用于数据清洗,它通过正则表达式去除name、age和gender字段中的非法字符,并将age字段转换为整数类型,并过滤掉非正数值。Reducer类用于去除重复数据。Driver类用于设置MapReduce作业的各种参数,包括输入路径、输出路径、Mapper类、Reducer类等。最终,执行Driver类的main方法即可启动MapReduce作业,完成数据清洗。
阅读全文
相关推荐
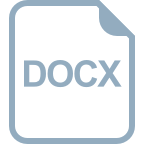
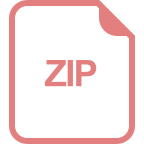
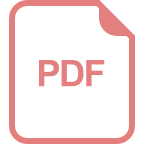
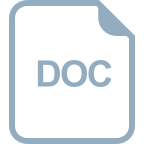
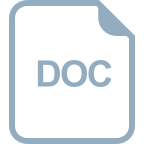

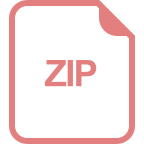
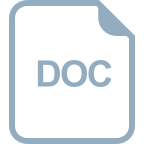
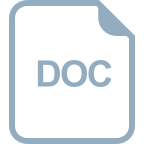
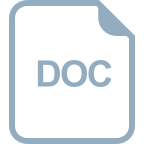
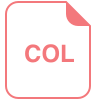
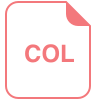
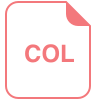
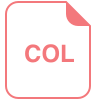
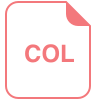