编写一个Java MapReduce程序来处理您提供的数据格式,并将其最后一列(时间戳)转换为日期格式(如2017-11-23),同时保持该行其他数据不变,Mapper、Reducer、Driver三个一个都不能被省略
时间: 2024-09-22 12:01:22 浏览: 49
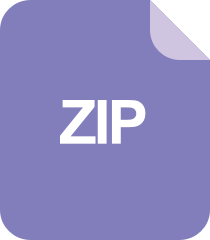
可视化毕业设计:数据处理(MapReduce)+数据展示(hbase+java+echarts).zip
为了处理给定的数据格式并实现所需的功能——将时间戳转换成指定的日期格式(例如“2017-11-23”),可以设计一个Java MapReduce程序。这个程序包括Mapper、Reducer以及驱动代码(Driver)。下面提供了一个概念性的框架:
### Mapper部分
Mapper的作用是从输入中提取出每一条记录,并对需要转换的时间戳进行处理。假设我们使用Hadoop的默认分隔符(逗号),Mapper类应该如下所示:
```java
import java.io.IOException;
import java.text.SimpleDateFormat;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public class DataFormatMapper extends Mapper<LongWritable, Text, Text, Text> {
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyyMMdd");
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] parts = value.toString().split(",");
if (parts.length > 0) {
// 将时间戳转换为日期格式
String dateString = dateFormat.format(Long.parseLong(parts[parts.length - 1]));
StringBuilder sb = new StringBuilder();
for (int i = 0; i < parts.length - 1; i++) {
sb.append(parts[i]).append(",");
}
sb.append(dateString);
context.write(new Text(), new Text(sb.toString()));
}
}
}
```
### Reducer部分
在这个场景下,Reducer主要是输出Mapper阶段的结果,因为不需要对键值对做进一步的聚合操作。
```java
import java.io.IOException;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public class DataFormatReducer extends Reducer<Text, Text, Text, Text> {
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
for (Text val : values) {
context.write(key, val);
}
}
}
```
### Driver部分
最后是Driver类,用于设置MapReduce作业的相关参数,并启动作业执行。
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class DataFormatDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "data format conversion");
job.setJarByClass(DataFormatDriver.class);
job.setMapperClass(DataFormatMapper.class);
job.setReducerClass(DataFormatReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
此方案提供了基本的结构和逻辑以完成任务要求。注意实际编码时还需要考虑异常处理和其他细节优化。
阅读全文
相关推荐
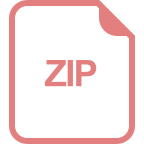
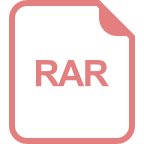

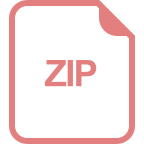
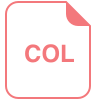
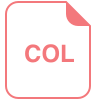
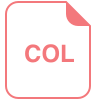
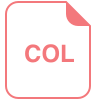
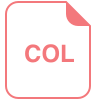
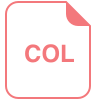
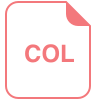
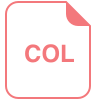
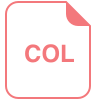
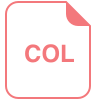
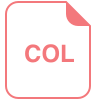
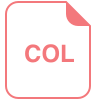
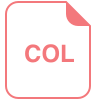
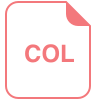