编写一个Java MapReduce程序来处理您提供的数据格式,并将其最后一列(时间戳)转换为日期格式(如2017-11-23),同时保持该行其他数据与切割好的时间一起保存下来
时间: 2024-09-21 13:09:34 浏览: 34
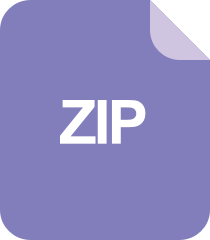
可视化毕业设计:数据处理(MapReduce)+数据展示(hbase+java+echarts).zip
为了处理给定的数据并实现所需的功能,我们可以设计一个简单的MapReduce程序。这个程序的目标是从原始的时间戳中提取日期部分,并且保留每行记录中的其它数据字段。下面提供了一个基本框架:
### Mapper
Mapper的作用是将输入键值对`<String, String>`转化为输出键值对`<(自定义键, 值)>`。在这个场景下,我们希望以处理后的时间作为键的一部分,并且保留整个行的信息作为值。
```java
import java.io.IOException;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public class DateExtractorMapper extends Mapper<LongWritable, Text, Text, Text> {
private static final Text outputKey = new Text();
private static final Text outputValue = new Text();
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// Split the line into individual elements based on commas.
String[] parts = value.toString().split(",");
if (parts.length >= 2) { // Ensure we have enough parts to work with.
// Extract the timestamp from the last column and convert it to date format "YYYY-MM-DD".
String timestamp = parts[parts.length - 1];
String formattedDate = convertTimestampToDate(timestamp); // Implement this function or use SimpleDateFormat.
StringBuilder builder = new StringBuilder();
for (int i = 0; i < parts.length - 1; i++) {
builder.append(parts[i]).append(",");
}
builder.deleteCharAt(builder.length() - 1); // Remove trailing comma.
outputKey.set(formattedDate);
outputValue.set(builder.toString());
context.write(outputKey, outputValue);
}
}
// Dummy implementation of converting timestamp to date. Should be replaced with actual logic.
private String convertTimestampToDate(String timestamp) {
return timestamp.substring(0, 8).replaceFirst("(\\d{4})(\\d{2})(\\d{2})", "$1-$2-$3");
}
}
```
### Reducer
Reducer可以在这个例子中被省略,因为我们只需要映射器来完成任务,即不进行进一步的数据聚合或减少操作。
以上代码展示了如何使用Hadoop的MapReduce API来解析每一行数据,并将最后的时间戳字段转化成所需的日期格式。注意这里简化了时间戳到日期的转换逻辑,实际应用时需要根据具体的时间戳格式采用合适的方法来实现这一功能。
阅读全文
相关推荐
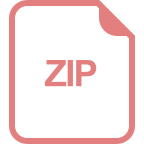
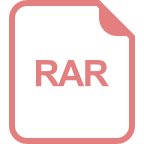
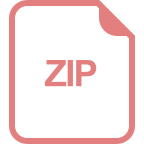
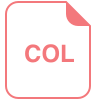
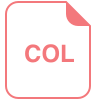
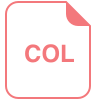
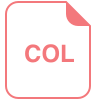
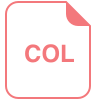
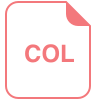
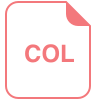
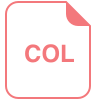
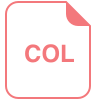
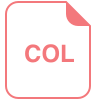
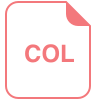
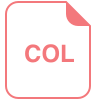
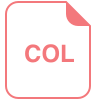
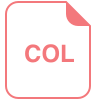
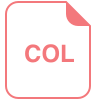