用VHDL语言设计一个4位抢答器。通过控制数码管实现倒计时显示,倒计时为9秒。显示为零后开始抢答,当有一路抢答按键按下,该路抢答信号将其余各路抢答信号封锁,并通过4个LED灯显示抢答结果(当1号选手抢答成功则灯A亮;当2号选手抢答成功则灯B亮;当3号选手抢答成功则灯C亮;当4号选手抢答成功则灯D亮;其他情况灯灭)。若在倒计时结束前抢答,则本选手成绩无效。
时间: 2023-12-31 15:03:45 浏览: 22
以下是一个简单的4位抢答器的VHDL代码,其中使用了一个计数器来实现倒计时,并且使用了一个状态机控制抢答过程。其中使用了7个数码管来显示倒计时,4个LED灯来显示抢答结果。
```
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity response_timer is
port(
key1: in std_logic; -- 抢答键1
key2: in std_logic; -- 抢答键2
key3: in std_logic; -- 抢答键3
key4: in std_logic; -- 抢答键4
clk: in std_logic; -- 时钟
reset: in std_logic; -- 复位
seg: out std_logic_vector(6 downto 0); -- 数码管
led: out std_logic_vector(3 downto 0) -- LED灯
);
end response_timer;
architecture rtl of response_timer is
signal count: unsigned(13 downto 0); -- 倒计时计数器
signal state: integer range 0 to 5; -- 状态机状态
signal response: std_logic_vector(3 downto 0); -- 抢答信号
begin
-- 数码管显示模块
seg_display: entity work.seven_segment_display
port map(
clk => clk,
reset => reset,
data => count(11 downto 8),
seg => seg
);
-- 倒计时计数器
count_process: process(clk, reset)
begin
if reset = '1' then
count <= (others => '0');
elsif rising_edge(clk) then
if count = 0 then
count <= std_logic_vector(to_unsigned(9999, count'length));
else
count <= count - 1;
end if;
end if;
end process;
-- 状态机
state_process: process(clk, reset)
begin
if reset = '1' then
state <= 0;
response <= (others => '0');
led <= (others => '0');
elsif rising_edge(clk) then
case state is
when 0 => -- 显示倒计时
if count = 0 then
state <= 1; -- 进入抢答状态
end if;
when 1 => -- 抢答状态
if key1 = '1' and response(0) = '0' then
response <= "0001";
state <= 2;
elsif key2 = '1' and response(1) = '0' then
response <= "0010";
state <= 2;
elsif key3 = '1' and response(2) = '0' then
response <= "0100";
state <= 2;
elsif key4 = '1' and response(3) = '0' then
response <= "1000";
state <= 2;
elsif count = 0 then
state <= 3; -- 进入结果显示状态
end if;
when 2 => -- 抢答成功
state <= 3; -- 进入结果显示状态
when 3 => -- 结果显示状态
if response = "0001" then
led <= "0001"; -- 选手1抢答成功
elsif response = "0010" then
led <= "0010"; -- 选手2抢答成功
elsif response = "0100" then
led <= "0100"; -- 选手3抢答成功
elsif response = "1000" then
led <= "1000"; -- 选手4抢答成功
else
led <= (others => '0'); -- 没有选手抢答成功
end if;
end case;
end if;
end process;
end rtl;
```
相关推荐
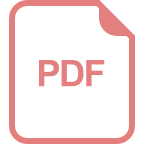
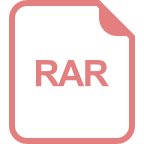














