请用Java图形界面的知识,编写一个图书管理系统,要求有管理界面。
时间: 2023-03-05 17:38:21 浏览: 105
好的,以下是一个简单的用Java图形界面编写的图书管理系统示例,具备基本的管理功能。
首先,我们需要创建一个包含以下功能的管理界面:
1. 添加图书
2. 删除图书
3. 修改图书信息
4. 搜索图书
5. 显示图书列表
我们可以使用Java的Swing库来创建这些界面,其中包括JFrame、JPanel、JLabel、JTextField、JButton等组件。下面是一个简单的例子:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class BookManagementSystem extends JFrame {
private JPanel controlPanel;
private JLabel titleLabel, authorLabel, yearLabel;
private JTextField titleText, authorText, yearText;
private JButton addButton, deleteButton, updateButton, searchButton, displayButton;
public BookManagementSystem() {
setTitle("图书管理系统");
setSize(400, 300);
controlPanel = new JPanel();
controlPanel.setLayout(new GridLayout(5, 2));
titleLabel = new JLabel("书名:");
authorLabel = new JLabel("作者:");
yearLabel = new JLabel("出版年份:");
titleText = new JTextField();
authorText = new JTextField();
yearText = new JTextField();
addButton = new JButton("添加");
deleteButton = new JButton("删除");
updateButton = new JButton("修改");
searchButton = new JButton("搜索");
displayButton = new JButton("显示");
controlPanel.add(titleLabel);
controlPanel.add(titleText);
controlPanel.add(authorLabel);
controlPanel.add(authorText);
controlPanel.add(yearLabel);
controlPanel.add(yearText);
controlPanel.add(addButton);
controlPanel.add(deleteButton);
controlPanel.add(updateButton);
controlPanel.add(searchButton);
controlPanel.add(displayButton);
add(controlPanel);
setVisible(true);
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 添加图书操作
}
});
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 删除图书操作
}
});
updateButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 修改图书信息操作
}
});
searchButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 搜索图书操作
}
});
displayButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// 显示图书列表操作
}
});
}
}
```
然后,我们需要实现添加、删除、修改、搜索和显示图书列表的方法,可以使用Java的IO库来存储和读取数据。下面是一个简单的例子:
```java
import java.io.*;
import java.util.ArrayList;
import java.util.List;
public class Book {
private String title;
private String author;
private int year;
public Book(String title, String author, int year) {
this.title = title;
this.author = author;
this.year = year;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author
相关推荐
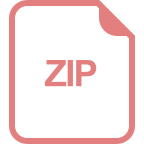














