CGAL点云区域生长代码
时间: 2023-09-11 15:10:39 浏览: 47
以下是一个使用CGAL库的点云区域生长算法的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <CGAL/Exact_predicates_inexact_constructions_kernel.h>
#include <CGAL/Point_set_3.h>
#include <CGAL/property_map.h>
#include <CGAL/Point_set_3/Point_set_processing_3.h>
typedef CGAL::Exact_predicates_inexact_constructions_kernel Kernel;
typedef Kernel::FT FT;
typedef Kernel::Point_3 Point;
typedef CGAL::Point_set_3<Point> Point_set;
int main()
{
// 读取点云数据
Point_set points;
std::ifstream stream("point_cloud.xyz");
if (!stream ||
!CGAL::read_xyz_points(stream, std::back_inserter(points))) {
std::cerr << "Error: cannot read file point_cloud.xyz" << std::endl;
return 1;
}
// 定义区域生长算法参数
std::vector<std::size_t> region;
std::vector<Point> seeds;
CGAL::parameters::PointPmap<Point_set> point_pmap(points);
CGAL::parameters::NormalPmap<Point_set> normal_pmap(points);
CGAL::parameters::SeedMap<std::vector<Point>::const_iterator> seed_map(seeds.begin(), seeds.end());
CGAL::parameters::KsearchParams k_params(10);
CGAL::parameters::RegionGrowing::Parameters<Kernel> parameters(0.1 /*angle threshold*/,
0.5 /*radius threshold*/,
100 /*minimum cluster size*/,
10000 /*maximum number of neighbors to consider*/,
10 /*number of threads*/,
point_pmap /*point property map*/,
normal_pmap /*normal property map*/,
seed_map /*seed property map*/,
k_params /*k search parameters*/);
// 执行区域生长算法
CGAL::cpp11::result_of<CGAL::Point_set_processing_3::Region_growing<Point_set>::type>::type
region_growing = CGAL::region_growing<Point_set>(points, parameters);
// 输出结果
std::cout << "Number of clusters: " << region_growing.size() << std::endl;
for (std::size_t i = 0; i < region_growing.size(); ++i) {
std::cout << "Cluster " << i << " size: " << region_growing[i].size() << std::endl;
}
return 0;
}
```
这里的输入点云数据需要以 .xyz 格式存储,每行包含一个点的 x、y、z 坐标。区域生长算法的具体参数可以根据需要进行调整。
相关推荐
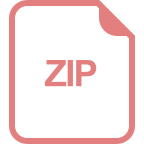














