c++使用zlib压缩文件夹
时间: 2023-07-27 09:07:06 浏览: 207
使用zlib库可以实现文件夹的压缩。下面是一个简单的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <zlib.h>
#include <dirent.h>
#define CHUNK_SIZE 16384
// 递归压缩文件夹
void compress(const std::string& folder_path, gzFile& output_file)
{
DIR* dir = opendir(folder_path.c_str());
if (dir == nullptr)
{
std::cerr << "Failed to open directory: " << folder_path << std::endl;
return;
}
dirent* entry;
while ((entry = readdir(dir)) != nullptr)
{
if (entry->d_type == DT_DIR)
{
// 忽略 . 和 .. 目录
if (std::string(entry->d_name) == "." || std::string(entry->d_name) == "..")
{
continue;
}
// 递归压缩子目录
std::string sub_folder_path = folder_path + "/" + entry->d_name;
compress(sub_folder_path, output_file);
}
else if (entry->d_type == DT_REG)
{
// 压缩文件
std::string file_path = folder_path + "/" + entry->d_name;
std::ifstream input_file(file_path, std::ios::binary);
if (!input_file.is_open())
{
std::cerr << "Failed to open file: " << file_path << std::endl;
continue;
}
char buffer[CHUNK_SIZE];
int ret;
while (input_file.read(buffer, CHUNK_SIZE))
{
ret = gzwrite(output_file, buffer, CHUNK_SIZE);
if (ret == 0)
{
std::cerr << "Failed to write data to output file: " << gzerror(output_file, &ret) << std::endl;
break;
}
}
if (input_file.gcount() > 0)
{
ret = gzwrite(output_file, buffer, input_file.gcount());
if (ret == 0)
{
std::cerr << "Failed to write data to output file: " << gzerror(output_file, &ret) << std::endl;
}
}
input_file.close();
}
}
closedir(dir);
}
int main()
{
std::string folder_path = "/path/to/folder";
std::string output_path = "/path/to/output.gz";
gzFile output_file = gzopen(output_path.c_str(), "wb");
if (output_file == nullptr)
{
std::cerr << "Failed to open output file: " << output_path << std::endl;
return 1;
}
compress(folder_path, output_file);
gzclose(output_file);
std::cout << "Compression completed successfully!" << std::endl;
return 0;
}
```
在上面的代码中,我们使用了 `opendir` 和 `readdir` 函数来遍历文件夹中的文件和子目录,使用 `std::ifstream` 读取文件内容,使用 `gzwrite` 将压缩后的数据写入到输出文件中。注意,在写入数据时要检查返回值,如果返回值为 0 则表示写入失败,可以使用 `gzerror` 获取错误信息。
需要注意的是,上面的代码只能压缩文件夹中的普通文件,不能压缩符号链接、设备文件等特殊文件。如果需要支持这些特殊文件,需要根据不同文件类型进行处理。
阅读全文
相关推荐
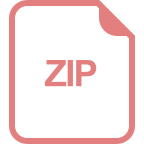

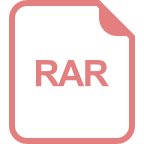
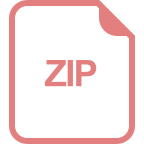

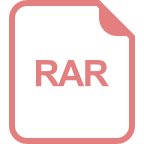
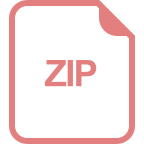
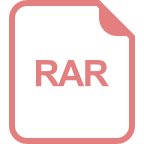



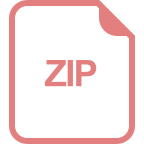
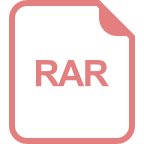
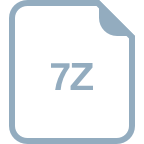
