使用uni-app写一个城市列表页面
时间: 2023-12-22 21:04:56 浏览: 36
好的,下面是一个简单的城市列表页面的代码示例:
```vue
<template>
<div class="city-list">
<div class="search-bar">
<input type="text" placeholder="请输入城市名称" v-model="searchText" @input="searchCity">
</div>
<div class="city-group" v-for="(cities, index) in cityList" :key="index">
<div class="group-title">{{ index }}</div>
<div class="city-item" v-for="(city, i) in cities" :key="i" @click="selectCity(city)">
{{ city }}
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
cityList: [], // 城市列表
searchText: '', // 搜索关键词
originalList: [], // 原始城市列表
};
},
mounted() {
this.initCityList();
},
methods: {
initCityList() {
// 初始化城市列表
// 这里可以根据需要从服务器获取城市数据
this.cityList = [
{
A: ['北京', '安徽', '澳门'],
},
{
B: ['保定', '包头', '巴彦淖尔'],
},
{
C: ['长沙', '成都', '重庆'],
},
{
D: ['大连', '东莞', '大理'],
},
{
E: ['鄂尔多斯', '恩施', '鄂州'],
},
{
F: ['福州', '阜阳', '佛山'],
},
{
G: ['广州', '贵阳', '桂林'],
},
{
H: ['杭州', '合肥', '哈尔滨'],
},
{
J: ['济南', '江门', '九江'],
},
{
K: ['昆明', '开封', '克拉玛依'],
},
{
L: ['丽江', '洛阳', '兰州'],
},
{
M: ['绵阳', '马鞍山', '眉山'],
},
{
N: ['南京', '南昌', '南宁'],
},
{
P: ['平顶山', '攀枝花', '莆田'],
},
{
Q: ['青岛', '泉州', '齐齐哈尔'],
},
{
S: ['上海', '深圳', '沈阳'],
},
{
T: ['天津', '太原', '台州'],
},
{
W: ['武汉', '无锡', '潍坊'],
},
{
X: ['西安', '厦门', '徐州'],
},
{
Y: ['烟台', '宜昌', '宜宾'],
},
{
Z: ['郑州', '珠海', '中山'],
},
];
// 将原始城市列表保存一份,方便搜索时使用
this.originalList = this.cityList.reduce((arr, group) => {
return arr.concat(Object.values(group)[0]);
}, []);
},
searchCity() {
// 搜索城市
if (this.searchText) {
const reg = new RegExp(this.searchText, 'i');
this.cityList = this.originalList.filter(city => reg.test(city));
} else {
// 搜索关键词为空时,显示所有城市
this.cityList = [
{
A: ['北京', '安徽', '澳门'],
},
{
B: ['保定', '包头', '巴彦淖尔'],
},
{
C: ['长沙', '成都', '重庆'],
},
{
D: ['大连', '东莞', '大理'],
},
{
E: ['鄂尔多斯', '恩施', '鄂州'],
},
{
F: ['福州', '阜阳', '佛山'],
},
{
G: ['广州', '贵阳', '桂林'],
},
{
H: ['杭州', '合肥', '哈尔滨'],
},
{
J: ['济南', '江门', '九江'],
},
{
K: ['昆明', '开封', '克拉玛依'],
},
{
L: ['丽江', '洛阳', '兰州'],
},
{
M: ['绵阳', '马鞍山', '眉山'],
},
{
N: ['南京', '南昌', '南宁'],
},
{
P: ['平顶山', '攀枝花', '莆田'],
},
{
Q: ['青岛', '泉州', '齐齐哈尔'],
},
{
S: ['上海', '深圳', '沈阳'],
},
{
T: ['天津', '太原', '台州'],
},
{
W: ['武汉', '无锡', '潍坊'],
},
{
X: ['西安', '厦门', '徐州'],
},
{
Y: ['烟台', '宜昌', '宜宾'],
},
{
Z: ['郑州', '珠海', '中山'],
},
];
}
},
selectCity(city) {
// 选择城市
// 这里可以根据需要将选择的城市传递给其他组件或页面
console.log(city);
},
},
};
</script>
<style scoped>
.city-list {
display: flex;
flex-direction: column;
align-items: center;
}
.search-bar {
width: 100%;
padding: 10px;
box-sizing: border-box;
}
.search-bar input {
width: 100%;
height: 40px;
border: 1px solid #ccc;
border-radius: 20px;
padding: 0 15px;
box-sizing: border-box;
background-color: #f5f5f5;
outline: none;
font-size: 16px;
}
.city-group {
width: 100%;
margin-top: 10px;
}
.group-title {
padding: 10px;
font-size: 18px;
font-weight: bold;
}
.city-item {
padding: 10px;
font-size: 16px;
cursor: pointer;
border-bottom: 1px solid #ccc;
transition: background-color 0.2s;
}
.city-item:last-child {
border-bottom: none;
}
.city-item:hover {
background-color: #f5f5f5;
}
</style>
```
这个页面实现了以下功能:
- 显示城市列表,按照拼音首字母分组显示
- 支持搜索城市,搜索结果会实时更新
- 支持选择城市,选择后会在控制台输出城市名称
注意:这个页面只是一个示例,实际项目中还需要根据具体需求进行优化和调整。
相关推荐
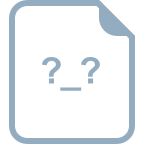
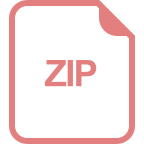
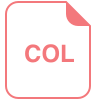
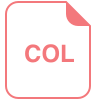
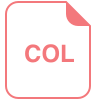
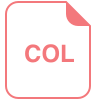
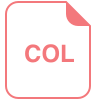










