请帮我写一个esp32连接阿里云的例程
时间: 2023-09-03 08:27:27 浏览: 95
### 回答1:
好的,以下是一个简单的esp32连接阿里云的例程:
#include <WiFi.h>
#include <PubSubClient.h>
// WiFi网络信息
const char* ssid = "你的WiFi名称";
const char* password = "你的WiFi密码";
// 阿里云物联网平台信息
const char* mqttServer = "你的MQTT服务器地址";
const int mqttPort = 1883;
const char* mqttUsername = "你的MQTT用户名";
const char* mqttPassword = "你的MQTT密码";
const char* mqttClientId = "你的MQTT客户端ID";
// WiFi客户端和MQTT客户端
WiFiClient wifiClient;
PubSubClient mqttClient(wifiClient);
void setup() {
// 初始化串口
Serial.begin(115200);
// 连接WiFi网络
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(100);
Serial.println("Connecting to WiFi...");
}
Serial.println("WiFi connected");
// 连接MQTT服务器
mqttClient.setServer(mqttServer, mqttPort);
mqttClient.setCallback(callback);
while (!mqttClient.connected()) {
if (mqttClient.connect(mqttClientId, mqttUsername, mqttPassword)) {
Serial.println("MQTT connected");
} else {
Serial.print("MQTT failed with state ");
Serial.println(mqttClient.state());
delay(200);
}
}
// 订阅主题
mqttClient.subscribe("你的订阅主题");
}
void loop() {
// 处理MQTT消息
mqttClient.loop();
// 发布消息
mqttClient.publish("你的发布主题", "你的消息内容");
}
void callback(char* topic, byte* payload, unsigned int length) {
// 处理接收到的消息
Serial.print("Message received: ");
Serial.println((char*)payload);
}
### 回答2:
esp32是一款强大的物联网开发板,在连接阿里云时,首先需要准备好以下步骤:
1. 注册阿里云账号并创建物联网平台实例。
2. 下载并安装ESP-IDF开发环境,ESP-IDF是官方提供的esp32开发框架,具有丰富的API和示例。
3. 在开发环境中创建一个新的工程。
下面是一个连接ESP32到阿里云的简单例程:
1. 首先,需要引入必要的库文件:
```c++
#include <stdio.h>
#include "esp_system.h"
#include "nvs_flash.h"
#include "wifi.h"
#include "aliyun_iot.h"
```
2. 初始化ESP系统和硬件:
```c++
void app_main()
{
// 初始化非易失性存储(NVS)
esp_err_t ret = nvs_flash_init();
if (ret == ESP_ERR_NVS_NO_FREE_PAGES || ret == ESP_ERR_NVS_NEW_VERSION_FOUND) {
ESP_ERROR_CHECK(nvs_flash_erase());
ret = nvs_flash_init();
}
ESP_ERROR_CHECK(ret);
// 初始化WiFi
wifi_init();
// 初始化阿里云IoT
aliyun_iot_init();
// 连接阿里云
aliyun_iot_connect();
}
```
3. 配置Wi-Fi网络:
```c++
void wifi_init()
{
// 配置Wi-Fi (注意:以下代码仅作示例,具体配置根据实际情况修改)
wifi_config_t wifi_config = {
.sta = {
.ssid = "YOUR_SSID",
.password = "YOUR_PASSWORD"
},
};
ESP_ERROR_CHECK(esp_wifi_set_mode(WIFI_MODE_STA));
ESP_ERROR_CHECK(esp_wifi_set_config(ESP_IF_WIFI_STA, &wifi_config));
ESP_ERROR_CHECK(esp_wifi_start());
}
```
4. 连接到阿里云:
```c++
void aliyun_iot_connect()
{
// 配置阿里云IoT连接信息(Endpoint、ProductKey、DeviceName、DeviceSecret)
aliyun_iot_config_t iot_config = {
.endpoint = "YOUR_ENDPOINT",
.product_key = "YOUR_PRODUCT_KEY",
.device_name = "YOUR_DEVICE_NAME",
.device_secret = "YOUR_DEVICE_SECRET"
};
// 连接到阿里云IoT
aliyun_iot_err_t err = aliyun_iot_connect(&iot_config);
if (err != ALIYUN_IOT_SUCCESS) {
printf("Failed to connect to Aliyun IoT, err: %d\n", err);
}
}
```
以上是一个简单的ESP32连接阿里云的例程,你可以根据实际情况修改Wi-Fi网络、阿里云IoT连接信息等设置。同时,你还可以在连接成功后,根据需要发送和接收数据,实现更丰富的物联网应用!
### 回答3:
ESP32是一款功能强大的物联网开发板,可以通过WiFi连接到互联网,并实现与云平台的通信。连接到阿里云的例程如下:
首先,在Arduino IDE中安装ESP32开发板支持库,然后打开一个新的Arduino项目。
然后,引入必要的库文件。在代码的开头,加上以下几行:
```
#include <WiFi.h>
#include <WiFiClientSecure.h>
#include <PubSubClient.h>
#include <ArduinoJson.h>
```
接下来,配置WiFi连接。在`setup`函数中,添加以下代码:
```
const char* ssid = "YOUR_SSID";
const char* password = "YOUR_PASSWORD";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
```
替换`YOUR_SSID`和`YOUR_PASSWORD`为你的WiFi网络名称和密码。
然后,配置阿里云IoT平台信息。在`setup`函数中,添加以下代码:
```
const char* aliyunServer = "YOUR_ALIYUN_SERVER";
const int aliyunPort = YOUR_ALIYUN_PORT;
const char* deviceId = "YOUR_DEVICE_ID";
const char* productKey = "YOUR_PRODUCT_KEY";
const char* deviceSecret = "YOUR_DEVICE_SECRET";
WiFiClientSecure client;
PubSubClient mqttClient(client);
void setup() {
// ...
mqttClient.setServer(aliyunServer, aliyunPort);
String clientId = String(deviceId) + "|securemode=2,signmethod=hmacsha1|";
mqttClient.setCallback(callback);
if (mqttClient.connect(clientId.c_str(), productKey, deviceSecret)) {
Serial.println("Connected to Aliyun IoT Platform");
} else {
Serial.println("Failed to connect to Aliyun IoT Platform");
}
mqttClient.subscribe("YOUR_TOPIC");
}
```
替换`YOUR_ALIYUN_SERVER`、`YOUR_ALIYUN_PORT`、`YOUR_DEVICE_ID`、`YOUR_PRODUCT_KEY`和`YOUR_DEVICE_SECRET`为你在阿里云平台中创建的设备信息。
最后,实现回调函数来处理接收到的消息:
```
void callback(char* topic, byte* payload, unsigned int length) {
Serial.print("Received message: ");
for (int i = 0; i < length; i++) {
Serial.print((char)payload[i]);
}
Serial.println();
}
```
以上是一个简单的ESP32连接阿里云的例程,可以实现基本的连接与消息接收。你可以根据自己的需求进一步扩展功能。
相关推荐
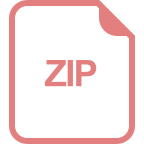
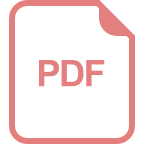














