请用java设计一个方法,复制一个文件到指定的文件夹
时间: 2024-04-08 19:35:32 浏览: 33
可以使用Java的文件操作类来实现文件复制功能。以下是一个示例方法:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileCopyExample {
public static void copyFile(String sourceFilePath, String destinationFolderPath) throws IOException {
// 创建源文件对象
File sourceFile = new File(sourceFilePath);
// 创建目标文件夹对象
File destinationFolder = new File(destinationFolderPath);
// 如果目标文件夹不存在,则创建
if (!destinationFolder.exists()) {
destinationFolder.mkdirs();
}
// 创建目标文件对象
File destinationFile = new File(destinationFolder, sourceFile.getName());
// 创建输入流和输出流
FileInputStream fis = new FileInputStream(sourceFile);
FileOutputStream fos = new FileOutputStream(destinationFile);
// 缓冲区大小(可根据实际情况调整)
byte[] buffer = new byte[1024];
int length;
// 逐个字节复制文件内容
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
// 关闭输入流和输出流
fis.close();
fos.close();
System.out.println("文件复制成功!");
}
public static void main(String[] args) throws IOException {
String sourceFilePath = "path/to/source/file.txt"; // 源文件路径
String destinationFolderPath = "path/to/destination/folder"; // 目标文件夹路径
copyFile(sourceFilePath, destinationFolderPath);
}
}
```
请将`sourceFilePath`和`destinationFolderPath`替换为实际的源文件路径和目标文件夹路径。调用`copyFile`方法即可完成文件复制操作。
相关推荐
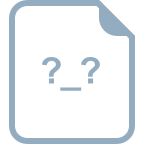














