用Java编程,把一个文件夹下所有的图片文件复制到指定文件夹下
时间: 2024-09-24 09:03:50 浏览: 47
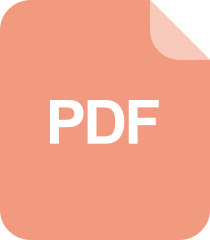
java递归实现复制一个文件夹下所有文件功能
在Java编程中,你可以使用`java.io.File`类以及`java.nio.file.Files`包下的功能来实现将一个文件夹下所有图片文件复制到另一个指定位置。以下是一个简单的示例:
```java
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class CopyImages {
public static void copyFolderToAnother(String sourceDirPath, String destinationDirPath) {
try {
// 获取源目录路径
Path source = Paths.get(sourceDirPath);
// 检查源目录是否存在并且是文件夹
if (!Files.isDirectory(source)) {
throw new IllegalArgumentException("Source directory does not exist or is not a folder.");
}
// 创建目标目录(如果不存在)
Files.createDirectories(Paths.get(destinationDirPath));
// 遍历源目录中的所有文件
for (Path entry : Files.newDirectoryStream(source)) {
// 检查是否为图像文件,假设我们只关心.jpg和.png
if (entry.toString().endsWith(".jpg") || entry.toString().endsWith(".png")) {
// 获取文件名和目标路径
String fileName = entry.getFileName().toString();
Path targetFile = Paths.get(destinationDirPath, fileName);
// 执行复制操作
Files.copy(entry, targetFile);
System.out.println("Copied file " + fileName + " to " + targetFile);
}
}
} catch (IOException e) {
System.err.println("Error copying files: " + e.getMessage());
}
}
public static void main(String[] args) {
String source = "/path/to/source/folder";
String destination = "/path/to/destination/folder";
copyFolderToAnother(source, destination);
}
}
```
在这个示例中,你需要替换`source`和`destination`变量为你实际的文件夹路径。这个脚本会查找并复制源目录下的所有`.jpg`和`.png`文件。
阅读全文
相关推荐
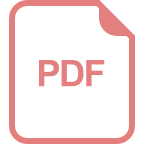
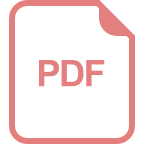
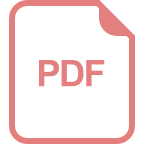
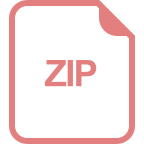
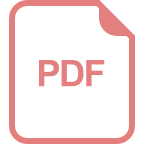
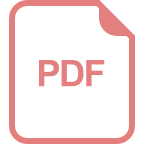











