怎么理解This line of code is searching for the index(position) of the substring "java" within the string "I love Java,java is very powerful!". It is case-sensitive, so it will only match the substring with lowercase 'j'. The result will depend on where the substring is found in the string. If it is found, the value of "index" will be the starting index of the first occurrence of the substring within the string. If the substring is not found, the value of "index" will be -1.
时间: 2024-04-27 16:25:05 浏览: 6
这段代码正在查找字符串"I love Java,java is very powerful!"中子字符串"java"的索引位置。它是大小写敏感的,因此只会匹配小写字母'j'的子字符串。结果将取决于子字符串在字符串中的位置。如果找到了子字符串,则"index"的值将是该子字符串在字符串中第一次出现的起始索引。如果未找到子字符串,则"index"的值将是-1。
相关问题
What is the correct description of a binary tree?
A binary tree is a tree data structure in which each node has at most two children, referred to as the left child and the right child. The left child node contains a value less than or equal to its parent node, while the right child node contains a value greater than or equal to its parent node. The nodes in a binary tree can be traversed in different ways such as in-order, pre-order, and post-order. Binary trees are commonly used in computer science for efficient data storage, searching, and sorting algorithms.
possible recursion found when searching for page 1 java 异常
这个错误通常发生在使用递归方法时,递归调用没有正确返回,导致程序进入无限循环的状态,最终导致程序崩溃。在Java中,递归方法的实现需要注意结束条件的设置,确保在满足结束条件时能够正确返回,否则会进入递归调用的无限循环中。解决这个错误的方法就是检查递归方法的实现,确保结束条件设置正确,并且在递归调用中能够正确返回,避免进入无限循环的状态。如果出现了这个错误,需要仔细检查代码逻辑,不断调试和修改代码,最终找到问题的根源并加以解决。同时,需要培养严谨的编程习惯,注意代码的规范性和可读性,减少出现这种错误的机会。
相关推荐
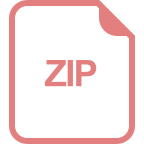
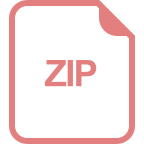












