使用Python编写一个界面,界面中能够显示动画,动画内容为,一个圆角矩形,它的边由若干个小矩形构成,这些小矩形沿着圆角矩形的边进行逆时针运动,请给出代码
时间: 2024-05-10 17:19:27 浏览: 8
可以使用Python的`pygame`库来实现这个界面,以下是一个简单的示例代码:
```python
import pygame
import math
pygame.init()
# 设置窗口大小和标题
size = (600, 400)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("Animated Rounded Rectangle")
# 设置圆角矩形的参数
rect_width = 200
rect_height = 100
rect_x = 200
rect_y = 150
rect_radius = 20
# 计算圆角矩形的边界点
points = []
for angle in range(0, 91):
x = rect_x + rect_radius - rect_radius * math.cos(angle * math.pi / 180)
y = rect_y + rect_radius - rect_radius * math.sin(angle * math.pi / 180)
points.append((x, y))
for angle in range(0, 91):
x = rect_x + rect_width - rect_radius + rect_radius * math.sin(angle * math.pi / 180)
y = rect_y + rect_radius - rect_radius * math.cos(angle * math.pi / 180)
points.append((x, y))
for angle in range(0, 91):
x = rect_x + rect_width - rect_radius + rect_radius * math.cos(angle * math.pi / 180)
y = rect_y + rect_height - rect_radius + rect_radius * math.sin(angle * math.pi / 180)
points.append((x, y))
for angle in range(0, 91):
x = rect_x + rect_radius - rect_radius * math.sin(angle * math.pi / 180)
y = rect_y + rect_height - rect_radius + rect_radius * math.cos(angle * math.pi / 180)
points.append((x, y))
# 设置小矩形的参数
rect_size = 10
rect_spacing = 5
rect_color = (255, 255, 255)
rect_speed = 2
# 初始化小矩形的位置和方向
rect_positions = []
rect_directions = []
for i in range(len(points) - 1):
x1, y1 = points[i]
x2, y2 = points[i+1]
distance = math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)
direction_x = (x2 - x1) / distance
direction_y = (y2 - y1) / distance
for j in range(int(distance / (rect_size + rect_spacing))):
x = x1 + j * (rect_size + rect_spacing) * direction_x
y = y1 + j * (rect_size + rect_spacing) * direction_y
rect_positions.append((x, y))
rect_directions.append((direction_x, direction_y))
# 开始主循环
done = False
clock = pygame.time.Clock()
while not done:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
# 更新小矩形的位置
for i in range(len(rect_positions)):
x, y = rect_positions[i]
dx, dy = rect_directions[i]
rect_positions[i] = (x + dx * rect_speed, y + dy * rect_speed)
# 绘制圆角矩形和小矩形
screen.fill((0, 0, 0))
pygame.draw.polygon(screen, (255, 255, 255), points)
for x, y in rect_positions:
pygame.draw.rect(screen, rect_color, (x, y, rect_size, rect_size))
# 更新屏幕
pygame.display.flip()
clock.tick(60)
# 退出程序
pygame.quit()
```
运行这个程序,将会看到一个带有动画的圆角矩形界面。你可以调整参数来改变圆角矩形的大小、小矩形的大小和速度等。
相关推荐
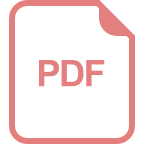
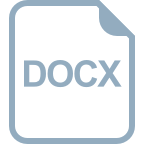
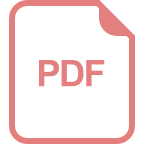














