用C语言写一个闭环收放卷程序
时间: 2023-10-01 10:11:33 浏览: 67
以下是一个简单的用C语言写的闭环收放卷程序的示例代码,仅供参考:
```c
#include <stdio.h>
#include <wiringPi.h>
#include <softPwm.h>
#define IN1_PIN 0 // IN1引脚
#define IN2_PIN 1 // IN2引脚
#define PWM_PIN IN1_PIN // PWM引脚
#define ENCODER_PIN 2 // 编码器引脚
#define MAX_SPEED 100 // 最大速度
#define MIN_SPEED 0 // 最小速度
#define MAX_COUNT 1000 // 最大计数值
#define MIN_COUNT 0 // 最小计数值
int count; // 当前计数值
int target_count = 500; // 目标计数值
int speed = 50; // 当前速度
// 初始化GPIO引脚和编码器
void init_pins() {
wiringPiSetup();
pinMode(IN1_PIN, OUTPUT);
pinMode(IN2_PIN, OUTPUT);
pinMode(ENCODER_PIN, INPUT);
softPwmCreate(PWM_PIN, 0, 100);
}
// 控制电机的转动方向和速度
void motor_control(int direction, int speed) {
if (direction == 1) {
digitalWrite(IN1_PIN, HIGH);
digitalWrite(IN2_PIN, LOW);
} else {
digitalWrite(IN1_PIN, LOW);
digitalWrite(IN2_PIN, HIGH);
}
softPwmWrite(PWM_PIN, speed);
}
// 读取编码器计数值
int read_encoder() {
int count = 0;
for (int i = 0; i < 20; i++) {
if (digitalRead(ENCODER_PIN) == HIGH) {
count++;
}
}
return count;
}
int main() {
init_pins(); // 初始化引脚和编码器
while (1) {
// 读取当前计数值
int current_count = read_encoder();
// 计算误差值
int error = target_count - current_count;
// 根据误差值调整速度
if (error > 0) {
speed++;
} else if (error < 0) {
speed--;
}
// 限制速度范围
if (speed > MAX_SPEED) {
speed = MAX_SPEED;
} else if (speed < MIN_SPEED) {
speed = MIN_SPEED;
}
// 控制电机转动
if (error != 0) {
int direction = error > 0 ? 1 : -1;
motor_control(direction, speed);
} else {
motor_control(1, 0);
}
// 限制计数范围
if (count > MAX_COUNT) {
count = MAX_COUNT;
} else if (count < MIN_COUNT) {
count = MIN_COUNT;
}
}
return 0;
}
```
该程序使用了wiringPi库和softPwm库来控制GPIO引脚和PWM信号,使用编码器来实现闭环控制。在程序中,通过读取编码器计数值和设定的目标计数值来计算误差值,然后根据误差值调整电机速度和方向,以实现闭环控制。程序中还包含了限制速度和计数范围的代码,以保证卷绕的稳定性和安全性。请注意,该程序仅为示例代码,实际应用中需要根据具体需求进行修改和调整。
阅读全文
相关推荐


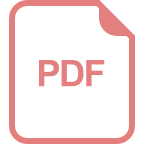
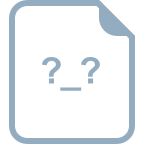
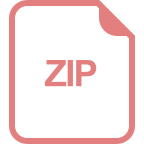
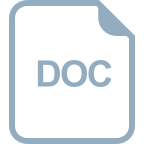
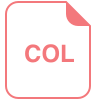
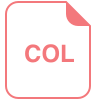
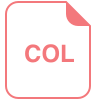
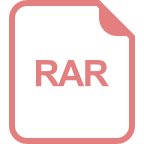
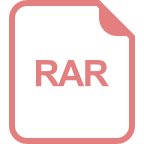
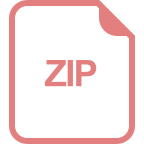
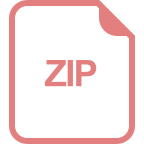