用Winhttp写一个POST https://sellercentral.amazon.com.au/ap/signin 的自定义函数,参数有请求头和请求数据,return响应头和响应内容
时间: 2024-01-14 21:04:45 浏览: 79
好的,我可以帮你编写一个Winhttp的POST请求函数。以下是示例代码:
```c++
#include <windows.h>
#include <winhttp.h>
#include <iostream>
#include <string>
#pragma comment(lib, "winhttp.lib")
std::string postRequest(std::string url, std::string headers, std::string postData)
{
HINTERNET hSession = NULL, hConnect = NULL, hRequest = NULL;
DWORD dwSize = 0;
DWORD dwDownloaded = 0;
LPSTR pszOutBuffer = NULL;
std::string response;
// Initialize WinHTTP.
hSession = WinHttpOpen(L"WinHTTP Example/1.0", WINHTTP_ACCESS_TYPE_DEFAULT_PROXY, WINHTTP_NO_PROXY_NAME, WINHTTP_NO_PROXY_BYPASS, 0);
if (!hSession)
{
goto cleanup;
}
// Specify an HTTP server.
hConnect = WinHttpConnect(hSession, L"sellercentral.amazon.com.au", INTERNET_DEFAULT_HTTPS_PORT, 0);
if (!hConnect)
{
goto cleanup;
}
// Create an HTTP request handle.
hRequest = WinHttpOpenRequest(hConnect, L"POST", L"/ap/signin", NULL, WINHTTP_NO_REFERER, WINHTTP_DEFAULT_ACCEPT_TYPES, WINHTTP_FLAG_SECURE);
if (!hRequest)
{
goto cleanup;
}
// Add request headers.
if (!WinHttpAddRequestHeaders(hRequest, headers.c_str(), headers.length(), WINHTTP_ADDREQ_FLAG_ADD))
{
goto cleanup;
}
// Send a request.
if (!WinHttpSendRequest(hRequest, WINHTTP_NO_ADDITIONAL_HEADERS, 0, (LPVOID)postData.c_str(), postData.length(), postData.length(), 0))
{
goto cleanup;
}
// End the request.
if (!WinHttpReceiveResponse(hRequest, NULL))
{
goto cleanup;
}
// Query headers size.
if (!WinHttpQueryHeaders(hRequest, WINHTTP_QUERY_RAW_HEADERS_CRLF, WINHTTP_HEADER_NAME_BY_INDEX, NULL, &dwSize, WINHTTP_NO_HEADER_INDEX))
{
goto cleanup;
}
else
{
// Allocate memory for the buffer.
pszOutBuffer = new char[dwSize];
if (!pszOutBuffer)
{
goto cleanup;
}
// Read the headers.
if (!WinHttpQueryHeaders(hRequest, WINHTTP_QUERY_RAW_HEADERS_CRLF, WINHTTP_HEADER_NAME_BY_INDEX, pszOutBuffer, &dwSize, WINHTTP_NO_HEADER_INDEX))
{
goto cleanup;
}
else
{
response.append(pszOutBuffer);
response.append("\r\n");
}
}
// Read the response.
do
{
dwSize = 0;
if (!WinHttpQueryDataAvailable(hRequest, &dwSize))
{
goto cleanup;
}
if (dwSize == 0)
{
break;
}
// Allocate space for the buffer.
pszOutBuffer = new char[dwSize + 1];
if (!pszOutBuffer)
{
goto cleanup;
}
// Read the data.
ZeroMemory(pszOutBuffer, dwSize + 1);
if (!WinHttpReadData(hRequest, (LPVOID)pszOutBuffer, dwSize, &dwDownloaded))
{
goto cleanup;
}
// Append the response to the buffer.
response.append(pszOutBuffer);
// Free the memory allocated to the buffer.
delete[] pszOutBuffer;
} while (dwSize > 0);
cleanup:
// Clean up the resources.
if (hRequest) WinHttpCloseHandle(hRequest);
if (hConnect) WinHttpCloseHandle(hConnect);
if (hSession) WinHttpCloseHandle(hSession);
if (pszOutBuffer) delete[] pszOutBuffer;
return response;
}
```
这个函数接受三个参数:
- `url`:请求的URL地址,这里是`https://sellercentral.amazon.com.au/ap/signin`。
- `headers`:请求头,可以自行构造需要的请求头。
- `postData`:请求数据,可以自行构造需要POST的数据。
函数返回的是一个字符串,其中包含了响应头和响应内容。你可以使用这个函数来向指定URL发送POST请求,并获取响应。
阅读全文
相关推荐
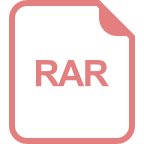
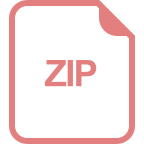









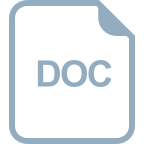





