使用drm的over_plane的示例C语言代码
时间: 2023-12-10 18:37:57 浏览: 244
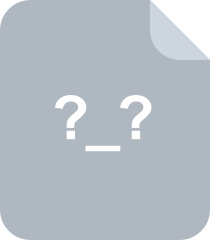
利用c写的C语言案例代码
以下是一个使用 DRM 的 `over_plane` 的示例 C 代码。它需要 Linux 系统和 DRM 库:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
#include <sys/mman.h>
#include <xf86drm.h>
#include <xf86drmMode.h>
#define BO_SIZE (4096 * 4096 * 4)
int main() {
int drm_fd = open("/dev/dri/card0", O_RDWR);
if (drm_fd < 0) {
fprintf(stderr, "Failed to open DRM device: %s\n", strerror(errno));
return 1;
}
// Get the primary plane id
drmModeRes *resources = drmModeGetResources(drm_fd);
if (!resources) {
fprintf(stderr, "Failed to get DRM resources: %s\n", strerror(errno));
close(drm_fd);
return 1;
}
int plane_id = -1;
for (int i = 0; i < resources->count_planes; i++) {
drmModePlane *plane = drmModeGetPlane(drm_fd, resources->planes[i]);
if (plane && (plane->possible_crtcs & (1 << 0))) {
plane_id = plane->plane_id;
drmModeFreePlane(plane);
break;
}
drmModeFreePlane(plane);
}
if (plane_id == -1) {
fprintf(stderr, "Failed to find primary plane\n");
drmModeFreeResources(resources);
close(drm_fd);
return 1;
}
// Create a buffer object
uint32_t handle;
int ret = drmPrimeHandleToFD(drm_fd, handle, 0, &handle);
if (ret < 0) {
fprintf(stderr, "Failed to prime handle: %s\n", strerror(errno));
drmModeFreeResources(resources);
close(drm_fd);
return 1;
}
uint32_t pitch = BO_SIZE / 4;
uint32_t bo_handles[4] = {handle, 0, 0, 0};
uint32_t bo_pitches[4] = {pitch, 0, 0, 0};
uint32_t bo_offsets[4] = {0, 0, 0, 0};
uint32_t bo_flags = 0;
uint32_t buffer_id;
ret = drmModeAddFB2(drm_fd, 4096, 4096, DRM_FORMAT_ARGB8888, bo_handles, bo_pitches, bo_offsets, &buffer_id, bo_flags);
if (ret < 0) {
fprintf(stderr, "Failed to create buffer object: %s\n", strerror(errno));
close(handle);
drmModeFreeResources(resources);
close(drm_fd);
return 1;
}
// Set the plane
drmModePlane *plane = drmModeGetPlane(drm_fd, plane_id);
if (!plane) {
fprintf(stderr, "Failed to get primary plane: %s\n", strerror(errno));
drmModeRmFB(drm_fd, buffer_id);
close(handle);
drmModeFreeResources(resources);
close(drm_fd);
return 1;
}
ret = drmModeSetPlane(drm_fd, plane_id, 0, 0, 0, 0, 0, 4096, 4096, 0, buffer_id, 0, 0, 0);
if (ret < 0) {
fprintf(stderr, "Failed to set plane: %s\n", strerror(errno));
drmModeFreePlane(plane);
drmModeRmFB(drm_fd, buffer_id);
close(handle);
drmModeFreeResources(resources);
close(drm_fd);
return 1;
}
drmModeFreePlane(plane);
// Wait for input
printf("Press Enter to exit...\n");
getchar();
// Clear the plane
ret = drmModeSetPlane(drm_fd, plane_id, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0);
if (ret < 0) {
fprintf(stderr, "Failed to clear plane: %s\n", strerror(errno));
}
// Cleanup
drmModeRmFB(drm_fd, buffer_id);
close(handle);
drmModeFreeResources(resources);
close(drm_fd);
return 0;
}
```
此代码将使用 DRM 在屏幕上显示一个空白的 4096x4096 像素的矩形,并等待用户按下回车键后退出。
阅读全文
相关推荐

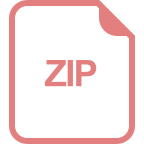
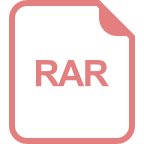
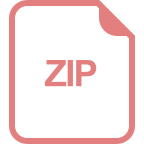
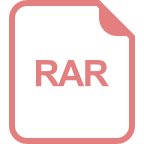

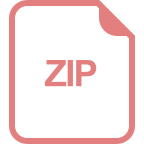
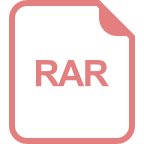
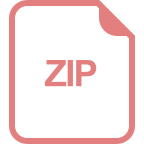
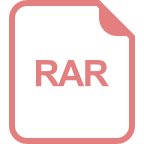
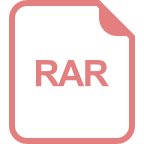
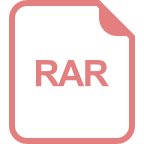
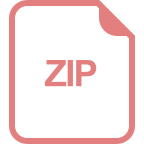

