用java代码实现银行家算法
时间: 2023-06-29 15:13:26 浏览: 82
以下是使用Java实现银行家算法的示例代码:
```java
import java.util.Arrays;
public class BankersAlgorithm {
private int[][] need; // 需求矩阵
private int[][] allocation; // 分配矩阵
private int[] available; // 可用资源向量
private int[] safeSequence; // 安全序列
public BankersAlgorithm(int[][] need, int[][] allocation, int[] available) {
this.need = need;
this.allocation = allocation;
this.available = available;
this.safeSequence = new int[need.length];
}
public boolean isSafe() {
boolean[] finish = new boolean[need.length]; // 标记进程是否完成
// 初始化工作
int[] work = Arrays.copyOf(available, available.length); // 复制可用资源向量
Arrays.fill(finish, false); // 将所有进程标记为未完成
// 安全检查
int count = 0; // 统计已完成的进程数
while (count < need.length) {
boolean found = false; // 是否找到符合条件的进程
for (int i = 0; i < need.length; i++) {
if (!finish[i] && Arrays.compare(need[i], work) <= 0) { // 进程未完成且需要的资源小于等于可用资源
work = add(work, allocation[i]); // 更新可用资源向量
safeSequence[count] = i; // 将进程添加到安全序列中
count++; // 已完成的进程数加一
finish[i] = true; // 标记该进程已完成
found = true; // 找到符合条件的进程
}
}
if (!found) { // 没有找到符合条件的进程,说明系统不安全
return false;
}
}
return true; // 所有进程都已完成,系统安全
}
public int[] getSafeSequence() {
return safeSequence;
}
private int[] add(int[] a, int[] b) { // 向量加法
int[] result = new int[a.length];
for (int i = 0; i < a.length; i++) {
result[i] = a[i] + b[i];
}
return result;
}
}
```
使用示例:
```java
int[][] need = {{3, 2, 1}, {0, 1, 0}, {1, 0, 2}, {0, 1, 1}, {2, 0, 0}}; // 需求矩阵
int[][] allocation = {{0, 0, 1}, {3, 0, 0}, {1, 0, 2}, {0, 1, 0}, {2, 0, 1}}; // 分配矩阵
int[] available = {3, 3, 2}; // 可用资源向量
BankersAlgorithm ba = new BankersAlgorithm(need, allocation, available);
if (ba.isSafe()) {
System.out.println("安全序列:" + Arrays.toString(ba.getSafeSequence()));
} else {
System.out.println("系统不安全!");
}
```
输出结果应为:
```
安全序列:[1, 3, 4, 0, 2]
```
阅读全文
相关推荐
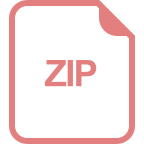
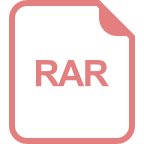
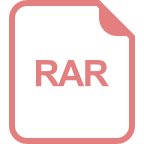
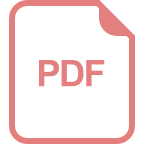
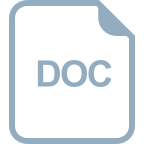
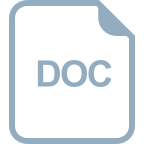
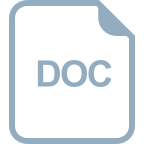




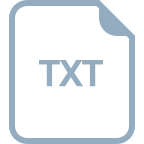