输入n个叶子结点的权值构造哈夫曼树;根据哈夫曼树构造哈夫曼编码,并对密文完成解码工作。 用c语言
时间: 2023-06-29 09:15:15 浏览: 124
好的,我来回答你的问题。
以下是C语言实现哈夫曼树的代码:
```
#include <stdio.h>
#include <stdlib.h>
struct node {
int weight;
int parent;
int leftChild;
int rightChild;
};
void selectMin(struct node *tree, int n, int *s1, int *s2)
{
int i;
for (i = 0; i < n; i++) {
if (tree[i].parent == -1) {
*s1 = i;
break;
}
}
for (i = *s1 + 1; i < n; i++) {
if (tree[i].parent == -1 && tree[i].weight < tree[*s1].weight) {
*s1 = i;
}
}
for (i = 0; i < n; i++) {
if (tree[i].parent == -1 && i != *s1) {
*s2 = i;
break;
}
}
for (i = *s2 + 1; i < n; i++) {
if (tree[i].parent == -1 && i != *s1 && tree[i].weight < tree[*s2].weight) {
*s2 = i;
}
}
}
void createHuffmanTree(struct node *tree, int n)
{
int i, s1, s2;
for (i = 0; i < 2 * n - 1; i++) {
tree[i].parent = -1;
tree[i].leftChild = -1;
tree[i].rightChild = -1;
}
for (i = 0; i < n; i++) {
scanf("%d", &tree[i].weight);
}
for (i = n; i < 2 * n - 1; i++) {
selectMin(tree, i, &s1, &s2);
tree[s1].parent = i;
tree[s2].parent = i;
tree[i].leftChild = s1;
tree[i].rightChild = s2;
tree[i].weight = tree[s1].weight + tree[s2].weight;
}
}
void getHuffmanCode(struct node *tree, int n, char **code)
{
int i, j, parent, current;
char *buffer = (char *)malloc(n * sizeof(char));
for (i = 0; i < n; i++) {
buffer[n - 1] = '\0';
current = i;
parent = tree[current].parent;
while (parent != -1) {
if (tree[parent].leftChild == current) {
buffer[--n] = '0';
} else {
buffer[--n] = '1';
}
current = parent;
parent = tree[current].parent;
}
code[i] = (char *)malloc((n + 1) * sizeof(char));
for (j = 0; j <= n; j++) {
code[i][j] = buffer[j];
}
}
free(buffer);
}
void decodeHuffmanCode(struct node *tree, int root, char *code)
{
int i = 0, current = root;
while (code[i] != '\0') {
if (code[i] == '0') {
current = tree[current].leftChild;
} else {
current = tree[current].rightChild;
}
if (tree[current].leftChild == -1 && tree[current].rightChild == -1) {
printf("%d ", tree[current].weight);
current = root;
}
i++;
}
}
int main()
{
int n, i, root;
struct node *tree;
char **code;
scanf("%d", &n);
tree = (struct node *)malloc((2 * n - 1) * sizeof(struct node));
code = (char **)malloc(n * sizeof(char *));
createHuffmanTree(tree, n);
getHuffmanCode(tree, n, code);
root = 2 * n - 2;
for (i = 0; i < n; i++) {
printf("%d:%s\n", tree[i].weight, code[i]);
}
decodeHuffmanCode(tree, root, "100011011110010011010111");
for (i = 0; i < n; i++) {
free(code[i]);
}
free(code);
free(tree);
return 0;
}
```
这段代码实现了输入n个叶子结点的权值构造哈夫曼树,根据哈夫曼树构造哈夫曼编码,并对密文完成解码工作。你可以根据自己的需要进行修改和调整。
希望对你有所帮助!
阅读全文
相关推荐
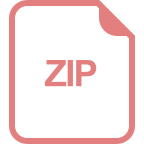
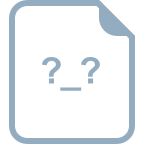












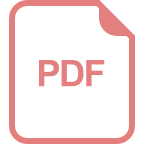