python简单通讯录系统
时间: 2023-10-09 13:06:25 浏览: 149
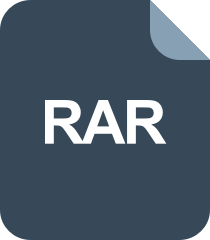
简单通讯录系统
以下是一个简单的 Python 通讯录系统,使用了字典和文件存储联系人信息:
```python
import os
# 从文件中读取联系人信息
def load_contacts():
if not os.path.exists("contacts.txt"):
return {}
with open("contacts.txt", "r") as f:
lines = f.readlines()
contacts = {}
for line in lines:
name, phone = line.strip().split(",")
contacts[name] = phone
return contacts
# 将联系人信息写入文件
def save_contacts(contacts):
with open("contacts.txt", "w") as f:
for name, phone in contacts.items():
f.write(f"{name},{phone}\n")
# 添加联系人
def add_contact(contacts):
name = input("请输入联系人姓名:")
phone = input("请输入联系人电话:")
if name in contacts:
print("联系人已存在")
else:
contacts[name] = phone
save_contacts(contacts)
print("联系人添加成功")
# 查找联系人
def search_contact(contacts):
name = input("请输入要查找的联系人姓名:")
if name in contacts:
print(f"{name} 的电话是 {contacts[name]}")
else:
print("联系人不存在")
# 删除联系人
def delete_contact(contacts):
name = input("请输入要删除的联系人姓名:")
if name in contacts:
del contacts[name]
save_contacts(contacts)
print("联系人已删除")
else:
print("联系人不存在")
# 显示所有联系人
def show_contacts(contacts):
if not contacts:
print("通讯录为空")
else:
print("所有联系人:")
for name, phone in contacts.items():
print(f"{name}:{phone}")
# 主程序
def main():
contacts = load_contacts()
while True:
print("1. 添加联系人")
print("2. 查找联系人")
print("3. 删除联系人")
print("4. 显示所有联系人")
print("5. 退出程序")
choice = input("请输入操作编号:")
if choice == "1":
add_contact(contacts)
elif choice == "2":
search_contact(contacts)
elif choice == "3":
delete_contact(contacts)
elif choice == "4":
show_contacts(contacts)
elif choice == "5":
break
else:
print("无效操作")
if __name__ == "__main__":
main()
```
该程序可以通过命令行界面进行操作,用户可以添加、查找、删除和显示所有联系人信息。联系人信息以文件的形式进行存储,每个联系人占据一行,姓名和电话号码用逗号分隔。
阅读全文
相关推荐
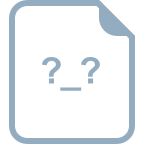


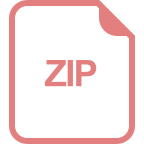
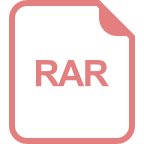
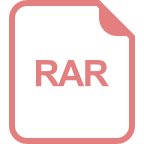









