#include <stdio.h> #include <stdlib.h> #include <time.h> typedef struct node { int data; struct node *next; } node; void insert(node **head, int value) { node *new_node = (node *)malloc(sizeof(node)); new_node->data = value; new_node->next = *head; *head = new_node; } void print(node *head) { while (head) { printf("%d ", head->data); head = head->next; } } void insertion_sort(node **head) { if (*head == NULL || (*head)->next == NULL) { return; } node *sorted_list = NULL; // 已排序部分的链表头指针 node *cur = *head; // 待排序部分的当前节点 while (cur != NULL) { node *prev_sorted = NULL; // 已排序部分的前一个节点 node *cur_sorted = sorted_list; // 已排序部分的当前节点 // 在已排序部分中找到待插入位置 while (cur_sorted != NULL && cur_sorted->data > cur->data) { prev_sorted = cur_sorted; cur_sorted = cur_sorted->next; } // 将待排序节点插入到已排序链表中 if (prev_sorted == NULL) { // 待插入位置在链表头 node *temp = cur->next; // 先保存下一个节点,以便后面遍历链表时继续访问 cur->next = sorted_list; sorted_list = cur; cur = temp; } else { // 待插入位置在链表中间或尾部 prev_sorted->next = cur; node *temp = cur->next; // 先保存下一个节点,以便后面遍历链表时继续访问 cur->next = cur_sorted; cur = temp; } } *head = sorted_list; // 更新头指针 } int main() { node *head = NULL; srand((unsigned int)time(0)); for (int i = 0; i < 10; ++i) { int a = rand() %100; insert(&head,a); } printf("原始链表:"); print(head); insertion_sort(&head); printf("\n排序后的链表:"); print(head); getchar(); return 0; }如何换成冒泡排序进行排序
时间: 2024-03-16 08:42:00 浏览: 59
要使用冒泡排序对链表进行排序,你需要将链表节点的值进行比较和交换。以下是一个基于冒泡排序的链表排序函数:
```
void bubble_sort(node **head) {
if (*head == NULL || (*head)->next == NULL) {
return;
}
int swapped; // 标记是否有交换发生
node *cur; // 当前节点
node *prev = NULL; // 前一个节点
do {
swapped = 0;
cur = *head;
while (cur->next != prev) {
if (cur->data > cur->next->data) {
int temp = cur->data;
cur->data = cur->next->data;
cur->next->data = temp;
swapped = 1;
}
cur = cur->next;
}
prev = cur;
} while (swapped);
}
```
在主函数中调用该函数即可完成链表的冒泡排序:
```
int main() {
node *head = NULL;
srand((unsigned int)time(0));
for (int i = 0; i < 10; ++i) {
int a = rand() %100;
insert(&head,a);
}
printf("原始链表:");
print(head);
bubble_sort(&head);
printf("\n排序后的链表:");
print(head);
getchar();
return 0;
}
```
阅读全文
相关推荐
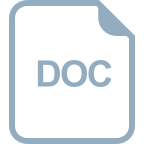
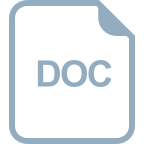
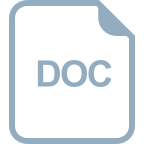





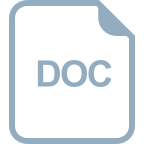










