用C++语言 设计一个类Salary用于统计一个员工的工资,其中使用一个静态数据成员sum salary保存工资总额和一个静态成员西数GetsalaryO返回该总工资。在主程序中定义Salary类的对象51和s2并初始化(学生自己指定实参值),,调用Getsalaryo西数输出运算结果。
时间: 2024-02-01 17:14:54 浏览: 26
以下是实现上述需求的C++代码:
```c++
#include <iostream>
using namespace std;
class Salary {
private:
static float sumSalary; // 静态数据成员,保存工资总额
float salary; // 员工的工资
public:
Salary(float s) { // 构造函数,初始化员工的工资
salary = s;
sumSalary += s;
}
static float GetSalaryO() { // 静态成员函数,返回总工资
return sumSalary;
}
};
float Salary::sumSalary = 0; // 静态数据成员初始化
int main() {
Salary s1(5000); // 创建对象s1,工资为5000
Salary s2(6000); // 创建对象s2,工资为6000
cout << "总工资为:" << Salary::GetSalaryO() << endl; // 调用静态成员函数输出总工资
return 0;
}
```
输出结果为:
```
总工资为:11000
```
相关问题
运用C++语言描述公司类、雇员类、职务类,每一个类应包含数据成员和成员函数。设计基类和派生类
以下是一个可能的实现示例:
```c++
// 公司类
class Company {
public:
Company(const std::string& name, const std::string& address) : name_(name), address_(address) {}
const std::string& GetName() const { return name_; }
const std::string& GetAddress() const { return address_; }
protected:
std::string name_;
std::string address_;
};
// 雇员职务类
class Job {
public:
Job(const std::string& title, double salary) : title_(title), salary_(salary) {}
const std::string& GetTitle() const { return title_; }
double GetSalary() const { return salary_; }
protected:
std::string title_;
double salary_;
};
// 雇员类,派生自公司类
class Employee : public Company {
public:
Employee(const std::string& name, const std::string& address, const std::string& id, const Job& job)
: Company(name, address), id_(id), job_(job) {}
const std::string& GetId() const { return id_; }
const Job& GetJob() const { return job_; }
protected:
std::string id_;
Job job_;
};
```
在此实现中,公司类包含名称和地址,而雇员类继承了公司类并添加了ID和职位。职务类包含职位标题和薪资。这个设计可能还有改进的空间,具体取决于应用场景和需求。
c++容器实现设计一个员工类 计算员工的薪资 公司有5个员工 按照员工工资排序,输出员工的工号
下面是一个使用 vector 容器实现的员工类和排序方法的示例代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class Employee {
public:
Employee(int id, double salary) : id(id), salary(salary) {}
int getId() const { return id; }
double getSalary() const { return salary; }
private:
int id;
double salary;
};
bool compareBySalary(const Employee& e1, const Employee& e2) {
return e1.getSalary() > e2.getSalary();
}
int main() {
vector<Employee> employees = {
Employee(1, 5000),
Employee(2, 8000),
Employee(3, 6000),
Employee(4, 7500),
Employee(5, 4500)
};
sort(employees.begin(), employees.end(), compareBySalary);
cout << "按照员工工资排序,输出员工的工号:" << endl;
for (const auto& employee : employees) {
cout << employee.getId() << endl;
}
return 0;
}
```
在上面的代码中,Employee 类有两个成员变量:id 和 salary,分别表示员工的工号和薪资。compareBySalary 函数是一个比较函数,用于按照员工工资进行排序。在 main 函数中,我们使用 vector 容器来存储 5 个员工对象,并按照员工工资进行排序,最后输出员工的工号。
相关推荐
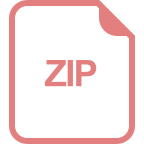
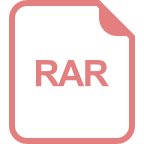
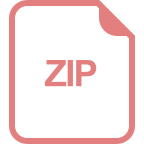












