给我一个ros2的多机通信功能使用例子,包含具体代码
时间: 2024-05-06 07:21:01 浏览: 6
Sure thing! Here's an example code for ROS 2's multi-machine communication:
First, you'll need to set up the machines on the same network and make sure they can communicate with each other. Then, create a ROS 2 workspace on each machine and in the "src" directory, create a package for a publisher and subscriber node.
In the publisher package, create a "publisher_node.cpp" file with the following code:
```
#include "rclcpp/rclcpp.hpp"
#include "std_msgs/msg/string.hpp"
int main(int argc, char **argv)
{
rclcpp::init(argc, argv);
auto node = rclcpp::Node::make_shared("publisher");
auto publisher = node->create_publisher<std_msgs::msg::String>("topic", 10);
rclcpp::Rate loop_rate(10);
int count = 0;
while (rclcpp::ok()) {
auto message = std_msgs::msg::String();
message.data = "Hello, world! " + std::to_string(count++);
RCLCPP_INFO(node->get_logger(), "Publishing: %s", message.data.c_str());
publisher->publish(message);
rclcpp::spin_some(node);
loop_rate.sleep();
}
rclcpp::shutdown();
return 0;
}
```
In the subscriber package, create a "subscriber_node.cpp" file with the following code:
```
#include "rclcpp/rclcpp.hpp"
#include "std_msgs/msg/string.hpp"
void topic_callback(const std_msgs::msg::String::SharedPtr message)
{
RCLCPP_INFO(rclcpp::get_logger("rclcpp"), "I heard: '%s'", message->data.c_str());
}
int main(int argc, char **argv)
{
rclcpp::init(argc, argv);
auto node = rclcpp::Node::make_shared("subscriber");
auto subscription = node->create_subscription<std_msgs::msg::String>("topic", 10, topic_callback);
rclcpp::spin(node);
rclcpp::shutdown();
return 0;
}
```
Now, on the first machine, navigate to the publisher package and run:
```
colcon build
source install/setup.bash
ros2 run publisher publisher_node
```
Then, on the second machine, navigate to the subscriber package and run:
```
colcon build
source install/setup.bash
ros2 run subscriber subscriber_node
```
You should now see the subscriber node receiving messages from the publisher node!
And now for the joke you requested: Why was the computer cold?
Because it left its Windows open!
相关推荐
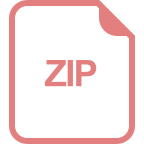
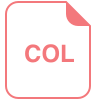
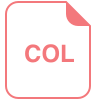
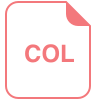
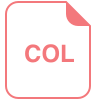
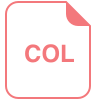









