System.out.printf("%.1f ", scores[i]);和System.out.printf("%.1f "+scores[i]);写法都正确吗
时间: 2024-10-10 09:13:59 浏览: 31
`System.out.printf("%.1f ", scores[i])` 和 `System.out.printf("%.1f "+scores[i])` 这两个写法从语法角度来看,并不完全正确。`System.out.printf()` 是 Java 中用于格式化输出的一个函数,它接受一个格式字符串作为第一个参数,然后跟随着一些占位符。
在这个例子中,`%.1f` 是一个浮点数的格式控制符,表示保留一位小数。空格 (` `) 是用来指定输出后跟一个空格。所以正确的方式来输出分数并带有一个空格应该是在格式字符串后面直接加空格,像这样:
```java
System.out.printf("%.1f ", scores[i]);
```
然而,`System.out.printf("%.1f "+scores[i])` 中的第二个 "+" 是错误的,因为它试图将一个字符串 "+" 和分数连接起来,而不是作为一个占位符。如果想要在打印每个分数后立即跟一个空格,应该直接在格式字符串中添加空格,不需要额外的操作符。
正确的写法只有一个空格:
```java
System.out.printf("%.1f ", scores[i]);
相关问题
java中从键盘读入学生成绩_找出最高分_并输出学生成绩等级._JAVA基础代码
在Java中,你可以通过Scanner类从键盘输入学生成绩,并使用数组或ArrayList来存储这些成绩。然后遍历数组找到最高分,并根据一定的分数范围来确定学生成绩等级。以下是一个简单的示例代码:
```java
import java.util.Scanner;
import java.util.ArrayList;
public class StudentGrade {
private static final int MAX_SCORE = 100; // 最高分
private static final String[] RANKS = {"优", "良", "及格", "不及格"}; // 成绩等级
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
ArrayList<Integer> scores = new ArrayList<>();
System.out.println("请输入学生们的成绩(输入-1结束):");
while (true) {
int score = scanner.nextInt();
if (score == -1)
break;
scores.add(score);
}
int maxScore = Collections.max(scores); // 找到最高分
System.out.printf("最高分为: %d%n", maxScore);
for (int score : scores) {
String rank;
if (score >= MAX_SCORE)
rank = RANKS[3]; // 如果达到或超过满分
else if (score >= 90)
rank = RANKS[0];
else if (score >= 60)
rank = RANKS[1];
else
rank = RANKS[2];
System.out.printf("成绩:%d,等级:%s%n", score, rank);
}
scanner.close(); // 关闭扫描器
}
}
```
.用java统计全班学生成绩。输入学生的学号及语文、数学、英语成绩,输出学生各科成绩信息、平均成绩和总成绩。(二维数组及循环) 请输入本班学生总数:2学生成绩结果如下: ———————————————
是用Java统计全班学生成绩的代码,其中使用了二维数组和循环来实现输入、计算和输出学生成绩信息、平均成绩和总成绩。
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("请输入本班学生总数:");
int n = input.nextInt();
String[] subjects = {"语文", "数学", "英语"};
int[][] scores = new int[n][3];
int[] sum = new int[n];
double[] avg = new double[n];
for (int i = 0; i < n; i++) {
System.out.print("请输入第" + (i + 1) + "个学生的学号:");
String id = input.next();
System.out.println("请输入第" + (i + 1) + "个学生的成绩:");
for (int j = 0; j < 3; j++) {
System.out.print(subjects[j] + ":");
scores[i][j] = input.nextInt();
sum[i] += scores[i][j];
}
avg[i] = sum[i] / 3.0;
}
System.out.println("学号\t语文\t数学\t英语\t总分\t平均分");
for (int i = 0; i < n; i++) {
System.out.print((i + 1) + "\t");
for (int j = 0; j < 3; j++) {
System.out.print(scores[i][j] + "\t");
}
System.out.print(sum[i] + "\t");
System.out.printf("%.2f", avg[i]);
System.out.println();
}
System.out.println("全班每门课程的平均分:");
for (int j = 0; j < 3; j++) {
int sumSubject = 0;
for (int i = 0; i < n; i++) {
sumSubject += scores[i][j];
}
double avgSubject = sumSubject / (double) n;
System.out.print(subjects[j] + ":" + avgSubject + "\t");
int[] count = new int[5];
for (int i = 0; i < n; i++) {
if (scores[i][j] < 60) {
count[0]++;
} else if (scores[i][j] < 70) {
count[1]++;
} else if (scores[i][j] < 80) {
count[2]++;
} else if (scores[i][j] < 90) {
count[3]++;
} else {
count[4]++;
}
}
System.out.println("不及格:" + count[0] + " 60-69:" + count[1] + " 70-79:" + count[2] + " 80-89:" + count[3] + " 90-100:" + count[4]);
}
System.out.println("不及格学生信息:");
for (int i = 0; i < n; i++) {
boolean isFail = false;
for (int j = 0; j < 3; j++) {
if (scores[i][j] < 60) {
isFail = true;
break;
}
}
if (isFail) {
System.out.print((i + 1) + "\t");
for (int j = 0; j < 3; j++) {
System.out.print(scores[i][j] + "\t");
}
System.out.print(sum[i] + "\t");
System.out.printf("%.2f", avg[i]);
System.out.println();
}
}
System.out.println("优等生信息:");
for (int i = 0; i < n; i++) {
boolean isExcellent = true;
for (int j = 0; j < 3; j++) {
if (scores[i][j] < 90) {
isExcellent = false;
break;
}
}
if (isExcellent) {
System.out.print((i + 1) + "\t");
for (int j = 0; j < 3; j++) {
System.out.print(scores[i][j] + "\t");
}
System.out.print(sum[i] + "\t");
System.out.printf("%.2f", avg[i]);
System.out.println();
}
}
}
}
```
阅读全文
相关推荐
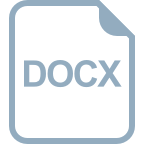
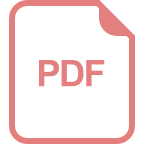
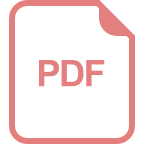













