某操作系统采用动态分区管理方法,用户区为512K,且始址为0,用空闲分区表管理空闲分区。若分配初始时,用户区空闲,且从低地址端开始分配,则对于下述请求序列,申请300K释放300K申请100K申请150K申请30K申请40K申请60K释放30K,分配内存时从低地址开始,C语言实现
时间: 2023-07-15 22:14:46 浏览: 104
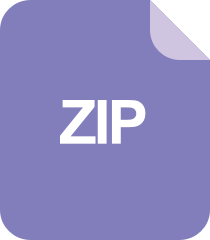
基于C语言实现操作系统内存管理【100010846】
以下是一个简单的C语言实现,使用空闲分区表管理空闲分区,动态分配和释放内存:
```c
#include <stdio.h>
#include <stdlib.h>
#define MEM_SIZE 512 // 内存大小
#define MIN_SIZE 5 // 最小可分配空间
#define FREE 0 // 空闲状态
#define ALLOC 1 // 分配状态
// 空闲分区表项
typedef struct free_block {
int size; // 分区大小
int start; // 起始地址
struct free_block *next; // 指向下一个空闲分区
} FreeBlock;
FreeBlock *free_list; // 空闲分区链表头指针
void init() {
free_list = (FreeBlock*)malloc(sizeof(FreeBlock)); // 初始化空闲分区链表
free_list->size = MEM_SIZE;
free_list->start = 0;
free_list->next = NULL;
}
int alloc_mem(int req_size) {
if (req_size <= 0) { // 请求大小非法
printf("Error: Invalid request size!\n");
return -1;
}
if (req_size < MIN_SIZE) { // 请求大小小于最小可分配空间
req_size = MIN_SIZE;
}
FreeBlock *p = free_list;
FreeBlock *prev = NULL;
while (p != NULL && p->size < req_size) { // 寻找第一个能满足请求的空闲分区
prev = p;
p = p->next;
}
if (p == NULL) { // 没有足够的空闲分区
printf("Error: Out of memory!\n");
return -1;
}
if (p->size == req_size) { // 正好能满足请求
p->size = ALLOC;
return p->start;
}
// 分割空闲分区
FreeBlock *new_block = (FreeBlock*)malloc(sizeof(FreeBlock));
new_block->size = req_size;
new_block->start = p->start;
new_block->next = p->next;
p->size -= req_size;
p->start += req_size;
if (prev == NULL) {
free_list = new_block;
} else {
prev->next = new_block;
}
new_block->size = ALLOC;
return new_block->start;
}
void free_mem(int start, int size) {
if (size <= 0) { // 释放大小非法
printf("Error: Invalid free size!\n");
return;
}
FreeBlock *p = free_list;
FreeBlock *prev = NULL;
while (p != NULL && p->start < start) { // 寻找被释放分区的前一个空闲分区
prev = p;
p = p->next;
}
if (prev == NULL) { // 被释放分区在链表头部
if (start + size == p->start) { // 合并空闲分区
p->start = start;
p->size += size;
} else { // 新增空闲分区
FreeBlock *new_block = (FreeBlock*)malloc(sizeof(FreeBlock));
new_block->size = size;
new_block->start = start;
new_block->next = p;
free_list = new_block;
}
} else if (p == NULL) { // 被释放分区在链表尾部
if (prev->start + prev->size == start) { // 合并空闲分区
prev->size += size;
} else { // 新增空闲分区
FreeBlock *new_block = (FreeBlock*)malloc(sizeof(FreeBlock));
new_block->size = size;
new_block->start = start;
new_block->next = NULL;
prev->next = new_block;
}
} else { // 被释放分区在链表中间
if (prev->start + prev->size == start && start + size == p->start) { // 合并空闲分区
prev->size += size + p->size;
prev->next = p->next;
free(p);
} else if (prev->start + prev->size == start) { // 合并前一个空闲分区
prev->size += size;
} else if (start + size == p->start) { // 合并后一个空闲分区
p->start = start;
p->size += size;
} else { // 新增空闲分区
FreeBlock *new_block = (FreeBlock*)malloc(sizeof(FreeBlock));
new_block->size = size;
new_block->start = start;
new_block->next = p;
prev->next = new_block;
}
}
}
void print_mem_state() {
printf("Memory state:\n");
FreeBlock *p = free_list;
while (p != NULL) {
printf("Free block: start=%d, size=%d\n", p->start, p->size);
p = p->next;
}
}
int main() {
init();
int p1 = alloc_mem(300);
print_mem_state();
int p2 = alloc_mem(300);
print_mem_state();
int p3 = alloc_mem(100);
print_mem_state();
int p4 = alloc_mem(150);
print_mem_state();
int p5 = alloc_mem(30);
print_mem_state();
int p6 = alloc_mem(40);
print_mem_state();
int p7 = alloc_mem(60);
print_mem_state();
free_mem(p5, 30);
print_mem_state();
return 0;
}
```
阅读全文
相关推荐
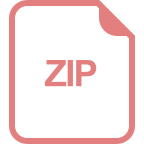

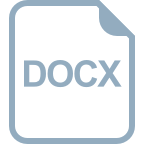
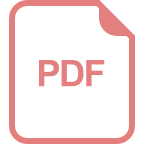
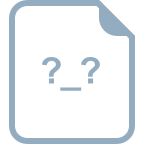
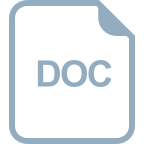
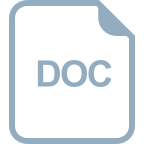



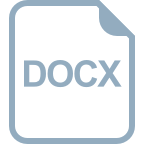
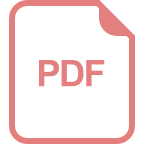
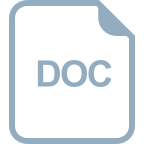
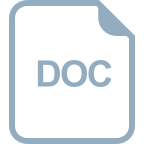
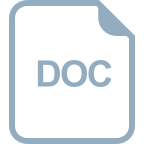
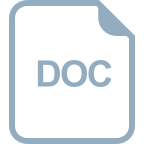
