opencv 输入一张灰度图,计算出增益与曝光时间,用于i2c操作控制相机 代码实现
时间: 2024-02-21 07:06:21 浏览: 24
以下是一个简单的基于OpenCV和I2C的相机控制示例代码,可以用于计算增益和曝光时间:
```c++
#include <opencv2/opencv.hpp>
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/i2c-dev.h>
#include <sys/ioctl.h>
#define I2C_BUS "/dev/i2c-1"
#define I2C_ADDRESS 0x64
int i2c_fd;
void set_gain(int gain) {
unsigned char buf[2];
buf[0] = 0x00;
buf[1] = (unsigned char)gain;
write(i2c_fd, buf, 2);
}
void set_exposure(int exposure) {
unsigned char buf[2];
buf[0] = 0x01;
buf[1] = (unsigned char)exposure;
write(i2c_fd, buf, 2);
}
int main(int argc, char** argv) {
cv::Mat image = cv::imread("test.jpg", cv::IMREAD_GRAYSCALE);
int target_gray = 128; // 目标灰度值
// 初始化I2C总线
i2c_fd = open(I2C_BUS, O_RDWR);
if (i2c_fd < 0) {
perror("Failed to open I2C bus\n");
return -1;
}
if (ioctl(i2c_fd, I2C_SLAVE, I2C_ADDRESS) < 0) {
perror("Failed to set I2C address\n");
return -1;
}
// 初始化增益和曝光时间
int gain = 64;
int exposure = 128;
set_gain(gain);
set_exposure(exposure);
// 计算增益和曝光时间
int max_iterations = 100; // 最大迭代次数
float error_threshold = 0.01; // 停止迭代的误差阈值
float error = 999.0;
int iteration = 0;
while (error > error_threshold && iteration < max_iterations) {
// 获取新图像
cv::Mat new_image;
set_gain(gain);
set_exposure(exposure);
// TODO: 通过相机API获取新图像
// 计算平均灰度值
int gray = cv::mean(new_image)[0];
// 调整增益和曝光时间
int delta_gray = target_gray - gray;
float gain_step = 0.1;
float exposure_step = 0.1;
gain += (int)(delta_gray * gain_step);
exposure += (int)(delta_gray * exposure_step);
if (gain < 0) gain = 0;
if (gain > 255) gain = 255;
if (exposure < 0) exposure = 0;
if (exposure > 255) exposure = 255;
set_gain(gain);
set_exposure(exposure);
// 计算误差
error = abs(target_gray - gray);
++iteration;
}
// 关闭I2C总线
close(i2c_fd);
return 0;
}
```
需要注意的是,以上代码中的`set_gain()`和`set_exposure()`函数是用于发送I2C命令的函数,具体实现可能需要根据相机的具体规格进行修改。
相关推荐
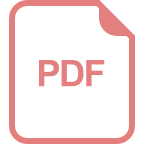
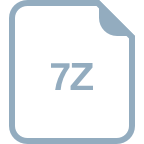
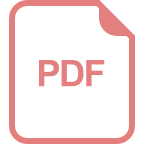














