用JS 写一个封装模态框,模态框有一个取消,删除,确认按钮,并有对应的事件
时间: 2024-03-27 18:40:08 浏览: 53
好的,以下是一个简单的JS封装模态框的代码,包含取消、删除和确认按钮,并添加了相应的事件监听器。
HTML部分:
```html
<!-- 模态框 -->
<div class="modal">
<div class="modal-content">
<p>确定要删除吗?</p>
<div class="modal-buttons">
<button id="cancel-btn">取消</button>
<button id="delete-btn">删除</button>
<button id="confirm-btn">确认</button>
</div>
</div>
</div>
<!-- 触发模态框的按钮 -->
<button id="show-modal-btn">显示模态框</button>
```
CSS部分:
```css
/* 遮罩层 */
.modal {
display: none;
position: fixed;
z-index: 100;
left: 0;
top: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
}
/* 模态框内容 */
.modal-content {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 20px;
background-color: #fff;
border-radius: 5px;
text-align: center;
}
/* 模态框按钮 */
.modal-buttons button {
margin: 0 10px;
padding: 5px 10px;
border-radius: 5px;
cursor: pointer;
}
/* 取消按钮 */
#cancel-btn {
background-color: #ccc;
color: #000;
}
/* 删除按钮 */
#delete-btn {
background-color: #f00;
color: #fff;
}
/* 确认按钮 */
#confirm-btn {
background-color: #0f0;
color: #fff;
}
```
JS部分:
```javascript
// 获取元素
const showModalBtn = document.getElementById("show-modal-btn");
const modal = document.querySelector(".modal");
const cancelBtn = document.getElementById("cancel-btn");
const deleteBtn = document.getElementById("delete-btn");
const confirmBtn = document.getElementById("confirm-btn");
// 显示模态框
showModalBtn.addEventListener("click", () => {
modal.style.display = "block";
});
// 隐藏模态框
function hideModal() {
modal.style.display = "none";
}
// 点击取消按钮
cancelBtn.addEventListener("click", () => {
hideModal();
});
// 点击删除按钮
deleteBtn.addEventListener("click", () => {
console.log("执行删除操作");
hideModal();
});
// 点击确认按钮
confirmBtn.addEventListener("click", () => {
console.log("执行确认操作");
hideModal();
});
```
这个模态框的基本功能已经实现了,你可以根据自己的需要进行进一步的定制。
阅读全文
相关推荐
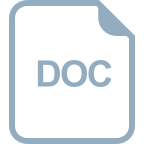
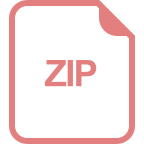
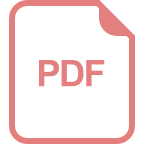
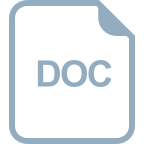
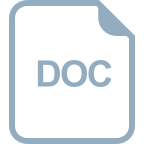
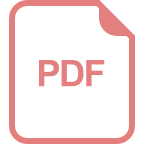
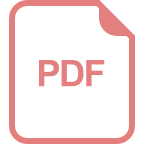
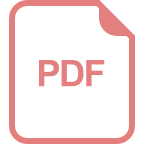
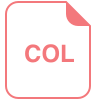
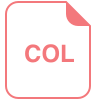
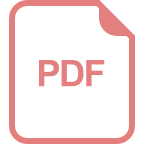
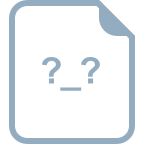
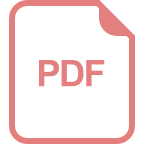
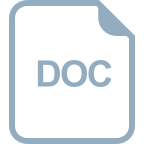

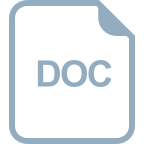