whoosh添加全文索引
时间: 2023-10-04 21:05:57 浏览: 42
要在Whoosh中添加全文索引,需要执行以下步骤:
1. 安装Whoosh:可以使用pip安装Whoosh。在命令行中运行以下命令:`pip install whoosh`
2. 创建索引:首先,您需要创建一个索引目录来存储索引。接下来,您需要定义一个Schema,它描述了要索引的文档的结构。示例代码:
```
from whoosh.fields import Schema, TEXT, ID
# 定义Schema,包含id和content两个字段
schema = Schema(id=ID(unique=True, stored=True), content=TEXT(stored=True))
```
3. 添加文档:使用Index对象将文档添加到索引中。示例代码:
```
from whoosh.index import create_in
from whoosh.index import open_dir
# 创建索引目录,如果已存在则打开
index_dir = "indexdir"
if not os.path.exists(index_dir):
os.mkdir(index_dir)
ix = create_in(index_dir, schema)
# ix = open_dir(index_dir) # 如果索引目录已存在,使用此行代码代替上一行代码打开索引
# 添加文档到索引
writer = ix.writer()
writer.add_document(id=u"1", content=u"This is the first document we've added!")
writer.add_document(id=u"2", content=u"The second one is even more interesting!")
writer.commit()
```
4. 搜索:使用QueryParser和Searcher对象执行搜索。示例代码:
```
from whoosh.qparser import QueryParser
# 创建查询解析器
qp = QueryParser("content", schema=schema)
# 创建查询对象
q = qp.parse("interesting")
# 执行查询
with ix.searcher() as searcher:
results = searcher.search(q)
print("Number of results:", len(results))
for hit in results:
print(hit["id"], hit["content"])
```
这是一个简单的Whoosh全文索引示例。您可以使用它作为起点,进一步扩展和优化搜索功能。
相关推荐
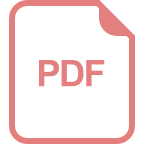
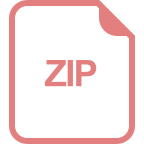















