图书管理 运用C++语言描述书籍类、图书类型类、图书类。设计基类和派生类,并运用多态性和虚函数的知识。要求设计具有继承与派生以及多态性的类。 要实现的主要功能如下: 1)建立书籍类、图书类型类、图书类 2)书籍、图书类型、图书信息的初始化 3)书籍、图书类型、图书信息的添加、修改、删除、查找 4)图书信息的输出 5)对图书信息按编号、书名排序 6)将图书信息保存为文件
时间: 2023-08-09 08:09:38 浏览: 46
好的,下面是一个简单的C++代码示例,实现了书籍类(Book)、图书类型类(BookType)、图书类(Library)以及对图书信息的添加、修改、删除、查找、排序和输出等功能。同时,使用了多态性和虚函数的知识。
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
#include <string>
using namespace std;
// 基类:书籍类
class Book {
protected:
string title;
string author;
string publisher;
int year;
int pages;
public:
Book(string t = "", string a = "", string p = "", int y = 0, int pg = 0)
: title(t), author(a), publisher(p), year(y), pages(pg) {}
virtual ~Book() {}
virtual void display() const = 0; // 纯虚函数
virtual void input() {
cout << "Title: ";
getline(cin, title);
cout << "Author: ";
getline(cin, author);
cout << "Publisher: ";
getline(cin, publisher);
cout << "Publication Year: ";
cin >> year;
cout << "Number of Pages: ";
cin >> pages;
cin.ignore(); // 忽略换行符
}
string getTitle() const {
return title;
}
string getAuthor() const {
return author;
}
string getPublisher() const {
return publisher;
}
int getYear() const {
return year;
}
int getPages() const {
return pages;
}
};
// 派生类:图书类型类
class BookType : public Book {
private:
string type;
public:
BookType(string t = "", string a = "", string p = "", int y = 0, int pg = 0, string tp = "")
: Book(t, a, p, y, pg), type(tp) {}
void display() const {
cout << "Title: " << title << endl;
cout << "Author: " << author << endl;
cout << "Publisher: " << publisher << endl;
cout << "Publication Year: " << year << endl;
cout << "Number of Pages: " << pages << endl;
cout << "Type: " << type << endl << endl;
}
void input() {
Book::input();
cout << "Type: ";
getline(cin, type);
}
string getType() const {
return type;
}
};
// 派生类:图书类
class Library : public Book {
private:
int id; // 图书编号
static int count; // 统计图书数目
public:
Library(string t = "", string a = "", string p = "", int y = 0, int pg = 0)
: Book(t, a, p, y, pg), id(++count) {}
void display() const {
cout << "ID: " << id << endl;
cout << "Title: " << title << endl;
cout << "Author: " << author << endl;
cout << "Publisher: " << publisher << endl;
cout << "Publication Year: " << year << endl;
cout << "Number of Pages: " << pages << endl << endl;
}
void input() {
Book::input();
}
int getID() const {
return id;
}
// 按编号排序
static bool cmpID(const Library& l1, const Library& l2) {
return l1.id < l2.id;
}
// 按书名排序
static bool cmpTitle(const Library& l1, const Library& l2) {
return l1.title < l2.title;
}
};
int Library::count = 0; // 初始化静态成员变量
// 图书管理类
class BookManager {
private:
vector<Book*> books; // 所有图书的指针数组
public:
~BookManager() {
for (int i = 0; i < books.size(); i++) {
delete books[i];
}
}
// 添加书籍
void addBook(Book* book) {
books.push_back(book);
}
// 修改书籍
void modifyBook(int id) {
for (int i = 0; i < books.size(); i++) {
Library* lib = dynamic_cast<Library*>(books[i]); // 动态类型转换
if (lib != nullptr && lib->getID() == id) {
lib->input();
cout << "Book modified successfully!" << endl << endl;
return;
}
}
cout << "Book not found!" << endl << endl;
}
// 删除书籍
void removeBook(int id) {
for (int i = 0; i < books.size(); i++) {
Library* lib = dynamic_cast<Library*>(books[i]);
if (lib != nullptr && lib->getID() == id) {
books.erase(books.begin() + i);
cout << "Book removed successfully!" << endl << endl;
return;
}
}
cout << "Book not found!" << endl << endl;
}
// 查找书籍
void searchBook(string keyword) {
for (int i = 0; i < books.size(); i++) {
Library* lib = dynamic_cast<Library*>(books[i]);
if (lib != nullptr && (lib->getTitle().find(keyword) != string::npos ||
lib->getAuthor().find(keyword) != string::npos)) {
books[i]->display();
}
}
}
// 按编号排序
void sortByID() {
sort(books.begin(), books.end(), Library::cmpID);
}
// 按书名排序
void sortByTitle() {
sort(books.begin(), books.end(), Library::cmpTitle);
}
// 输出所有图书信息
void displayAll() {
for (int i = 0; i < books.size(); i++) {
books[i]->display();
}
}
// 保存图书信息到文件
void saveToFile(string filename) {
ofstream fout(filename);
if (!fout) {
cout << "Failed to open file: " << filename << endl;
return;
}
for (int i = 0; i < books.size(); i++) {
fout << books[i]->getTitle() << '\t' << books[i]->getAuthor() << '\t'
<< books[i]->getPublisher() << '\t' << books[i]->getYear() << '\t'
<< books[i]->getPages() << '\t';
Library* lib = dynamic_cast<Library*>(books[i]);
if (lib != nullptr) {
fout << lib->getID();
}
fout << endl;
}
fout.close();
cout << "Books saved to file: " << filename << endl << endl;
}
};
int main() {
BookManager manager;
while (true) {
cout << "1. Add Book" << endl;
cout << "2. Modify Book" << endl;
cout << "3. Remove Book" << endl;
cout << "4. Search Book" << endl;
cout << "5. Sort by ID" << endl;
cout << "6. Sort by Title" << endl;
cout << "7. Display All Books" << endl;
cout << "8. Save to File" << endl;
cout << "0. Exit" << endl;
int choice;
cin >> choice;
cin.ignore(); // 忽略换行符
cout << endl;
switch (choice) {
case 1: {
cout << "1. Book Type" << endl;
cout << "2. Library" << endl;
int type;
cin >> type;
cin.ignore();
if (type == 1) {
BookType* book = new BookType();
book->input();
manager.addBook(book);
} else if (type == 2) {
Library* book = new Library();
book->input();
manager.addBook(book);
} else {
cout << "Invalid choice!" << endl << endl;
}
break;
}
case 2: {
int id;
cout << "Enter Book ID: ";
cin >> id;
manager.modifyBook(id);
break;
}
case 3: {
int id;
cout << "Enter Book ID: ";
cin >> id;
manager.removeBook(id);
break;
}
case 4: {
string keyword;
cout << "Enter Keyword: ";
getline(cin, keyword);
manager.searchBook(keyword);
break;
}
case 5: {
manager.sortByID();
cout << "Books sorted by ID." << endl << endl;
break;
}
case 6: {
manager.sortByTitle();
cout << "Books sorted by Title." << endl << endl;
break;
}
case 7: {
manager.displayAll();
break;
}
case 8: {
string filename;
cout << "Enter Filename: ";
getline(cin, filename);
manager.saveToFile(filename);
break;
}
case 0: {
return 0;
}
default: {
cout << "Invalid choice!" << endl << endl;
break;
}
}
}
}
```
该程序通过使用基类和派生类,实现了对书籍信息的建立,图书类型类和图书类继承自书籍类,同时使用了多态性和虚函数的知识,其中图书类(Library)还添加了一个静态成员变量count,统计图书数目。程序实现了对图书信息的初始化、添加、修改、删除、查找、排序和输出等功能,并可以将图书信息保存到文件中。
相关推荐
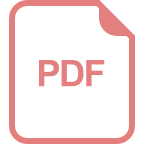
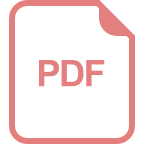
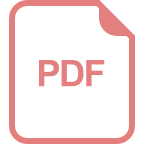











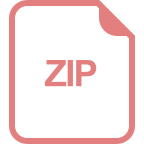
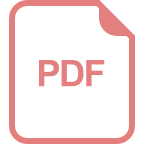
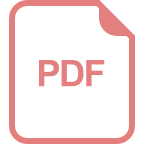
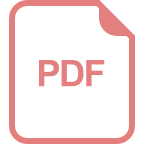
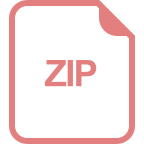