运用C++语言描述书籍类、图书类型类、图书类。设计基类和派生类。要求设计具有继承与派生以及多态性的类。帮我编写一个图书管理系统,具有这些功能: 1.建立书籍类、图书类型类、图书类 2.书籍、图书类型、图书信息的初始化 3.书籍、图书类型、图书信息的添加、修改、删除、查找 4.图书信息的输出 5.对图书信息按编号、书名排序 6.将图书信息保存为文件 7.读入文件信息
时间: 2023-08-11 17:08:38 浏览: 65
好的,我会根据你的要求编写一个C++的图书管理系统。首先,我们来设计基类和派生类:
```cpp
// 书籍类
class Book {
public:
Book(int id, std::string name, std::string author, std::string publisher, int year, int type_id);
int get_id();
std::string get_name();
std::string get_author();
std::string get_publisher();
int get_year();
int get_type_id();
protected:
int id_; // 书籍编号
std::string name_; // 书籍名称
std::string author_; // 作者
std::string publisher_; // 出版社
int year_; // 出版年份
int type_id_; // 图书类型编号
};
// 图书类型类
class BookType {
public:
BookType(int id, std::string name);
int get_id();
std::string get_name();
protected:
int id_; // 图书类型编号
std::string name_; // 图书类型名称
};
// 图书类
class Library {
public:
Library();
~Library();
void add_book(int id, std::string name, std::string author, std::string publisher, int year, int type_id);
void delete_book(int id);
void modify_book(int id, std::string name, std::string author, std::string publisher, int year, int type_id);
Book* find_book(int id);
void sort_by_id();
void sort_by_name();
void output_books();
void save_to_file(std::string file_name);
void read_from_file(std::string file_name);
private:
std::vector<Book*> books_; // 存储书籍的容器
std::vector<BookType*> types_; // 存储图书类型的容器
};
```
接下来,我们来实现这些类的具体功能:
```cpp
// 书籍类实现
Book::Book(int id, std::string name, std::string author, std::string publisher, int year, int type_id) {
id_ = id;
name_ = name;
author_ = author;
publisher_ = publisher;
year_ = year;
type_id_ = type_id;
}
int Book::get_id() {
return id_;
}
std::string Book::get_name() {
return name_;
}
std::string Book::get_author() {
return author_;
}
std::string Book::get_publisher() {
return publisher_;
}
int Book::get_year() {
return year_;
}
int Book::get_type_id() {
return type_id_;
}
// 图书类型类实现
BookType::BookType(int id, std::string name) {
id_ = id;
name_ = name;
}
int BookType::get_id() {
return id_;
}
std::string BookType::get_name() {
return name_;
}
// 图书类实现
Library::Library() {}
Library::~Library() {
for (auto book : books_) {
delete book;
}
for (auto type : types_) {
delete type;
}
}
void Library::add_book(int id, std::string name, std::string author, std::string publisher, int year, int type_id) {
// 检查书籍是否已经存在
for (auto book : books_) {
if (book->get_id() == id) {
std::cout << "该书籍已经存在" << std::endl;
return;
}
}
// 检查图书类型是否已经存在
bool type_exist = false;
for (auto type : types_) {
if (type->get_id() == type_id) {
type_exist = true;
break;
}
}
if (!type_exist) {
std::cout << "图书类型不存在" << std::endl;
return;
}
// 添加新书籍
Book* book = new Book(id, name, author, publisher, year, type_id);
books_.push_back(book);
}
void Library::delete_book(int id) {
for (auto it = books_.begin(); it != books_.end(); ++it) {
if ((*it)->get_id() == id) {
delete *it;
books_.erase(it);
return;
}
}
std::cout << "该书籍不存在" << std::endl;
}
void Library::modify_book(int id, std::string name, std::string author, std::string publisher, int year, int type_id) {
for (auto book : books_) {
if (book->get_id() == id) {
book->name_ = name;
book->author_ = author;
book->publisher_ = publisher;
book->year_ = year;
book->type_id_ = type_id;
return;
}
}
std::cout << "该书籍不存在" << std::endl;
}
Book* Library::find_book(int id) {
for (auto book : books_) {
if (book->get_id() == id) {
return book;
}
}
return nullptr;
}
void Library::sort_by_id() {
std::sort(books_.begin(), books_.end(), [](Book* a, Book* b) {
return a->get_id() < b->get_id();
});
}
void Library::sort_by_name() {
std::sort(books_.begin(), books_.end(), [](Book* a, Book* b) {
return a->get_name() < b->get_name();
});
}
void Library::output_books() {
for (auto book : books_) {
std::cout << "编号:" << book->get_id() << " 书名:" << book->get_name() << " 作者:" << book->get_author() << " 出版社:" << book->get_publisher() << " 出版年份:" << book->get_year() << " 类型编号:" << book->get_type_id() << std::endl;
}
}
void Library::save_to_file(std::string file_name) {
std::ofstream out(file_name);
for (auto book : books_) {
out << book->get_id() << " " << book->get_name() << " " << book->get_author() << " " << book->get_publisher() << " " << book->get_year() << " " << book->get_type_id() << std::endl;
}
out.close();
}
void Library::read_from_file(std::string file_name) {
std::ifstream in(file_name);
if (!in.is_open()) {
std::cout << "打开文件失败" << std::endl;
return;
}
int id, year, type_id;
std::string name, author, publisher;
while (in >> id >> name >> author >> publisher >> year >> type_id) {
add_book(id, name, author, publisher, year, type_id);
}
in.close();
}
```
最后,我们来编写一个main函数,测试图书管理系统的功能:
```cpp
int main() {
Library lib;
lib.add_book(1001, "C++ Primer", "Lippman", "机械工业出版社", 2005, 1);
lib.add_book(1002, "STL源码剖析", "侯捷", "电子工业出版社", 2006, 1);
lib.add_book(1003, "数据结构与算法分析", "Weiss", "清华大学出版社", 2012, 2);
lib.add_book(1004, "计算机网络", "谢希仁", "电子工业出版社", 2013, 3);
lib.add_book(1005, "深入理解计算机系统", "Bryant", "机械工业出版社", 2006, 4);
std::cout << "按编号排序:" << std::endl;
lib.sort_by_id();
lib.output_books();
std::cout << "按书名排序:" << std::endl;
lib.sort_by_name();
lib.output_books();
std::cout << "删除编号为1003的书籍" << std::endl;
lib.delete_book(1003);
std::cout << "修改编号为1002的书籍信息" << std::endl;
lib.modify_book(1002, "STL源码剖析", "侯捷", "清华大学出版社", 2006, 2);
std::cout << "查找编号为1001的书籍信息" << std::endl;
Book* book = lib.find_book(1001);
if (book) {
std::cout << "编号:" << book->get_id() << " 书名:" << book->get_name() << " 作者:" << book->get_author() << " 出版社:" << book->get_publisher() << " 出版年份:" << book->get_year() << " 类型编号:" << book->get_type_id() << std::endl;
} else {
std::cout << "该书籍不存在" << std::endl;
}
std::cout << "保存图书信息到文件book.txt" << std::endl;
lib.save_to_file("book.txt");
std::cout << "从文件book.txt读入图书信息" << std::endl;
lib.read_from_file("book.txt");
return 0;
}
```
这样,一个简单的图书管理系统就完成了。
相关推荐
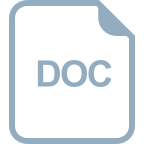










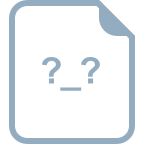
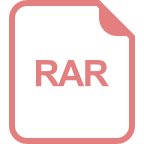
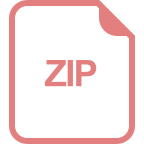
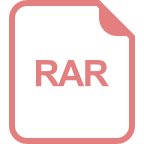
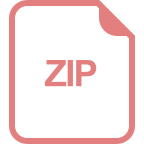
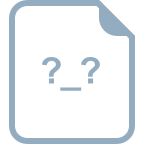