用c++实现点到平面的距离计算
时间: 2023-05-30 10:04:17 浏览: 89
假设有点P(x0, y0, z0)和平面ax + by + cz + d = 0,则点P到平面的距离为:
distance = |ax0 + by0 + cz0 + d| / sqrt(a^2 + b^2 + c^2)
可以使用下面的C代码来计算点到平面的距离:
#include <stdio.h>
#include <math.h>
typedef struct {
double x;
double y;
double z;
} Point3D;
typedef struct {
double a;
double b;
double c;
double d;
} Plane;
double distance(Point3D p, Plane plane) {
double numerator = plane.a * p.x + plane.b * p.y + plane.c * p.z + plane.d;
double denominator = sqrt(plane.a * plane.a + plane.b * plane.b + plane.c * plane.c);
return fabs(numerator / denominator);
}
int main() {
Point3D p = {1, 2, 3};
Plane plane = {1, 2, 3, -6};
double dist = distance(p, plane);
printf("Distance between point (%.2f, %.2f, %.2f) and plane (%.2fx + %.2fy + %.2fz + %.2f = 0) is %.2f\n",
p.x, p.y, p.z, plane.a, plane.b, plane.c, plane.d, dist);
return 0;
}
相关推荐
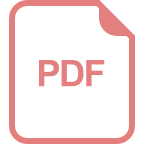
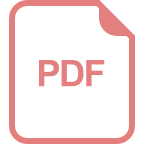













