用c语言编程实现生产者-消费者问题的模拟,要满足3点,(1)生产者消费者对缓冲区进行互斥操作。 (2)缓冲区大小为10,缓冲区满则不允许生产者生产数据,缓冲区空则不允许消费者消费数据。 (3)生产者消费者各循环操作10次
时间: 2023-05-26 15:07:15 浏览: 61
代码如下:
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int in = 0;
int out = 0;
int count = 0;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t full = PTHREAD_COND_INITIALIZER;
pthread_cond_t empty = PTHREAD_COND_INITIALIZER;
void *producer(void *arg)
{
int i, j;
int *id = (int *)arg;
for (i = 0; i < 10; i++) {
for (j = 0; j < 10; j++) {
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) {
printf("producer %d is waiting because buffer is full\n", *id);
pthread_cond_wait(&full, &mutex);
}
buffer[in] = rand() % 100;
printf("producer %d puts %d at buffer[%d]\n", *id, buffer[in], in);
in = (in + 1) % BUFFER_SIZE;
count++;
pthread_cond_signal(&empty);
pthread_mutex_unlock(&mutex);
usleep(rand() % 1000);
}
}
pthread_exit(NULL);
}
void *consumer(void *arg)
{
int i, j;
int *id = (int *)arg;
for (i = 0; i < 10; i++) {
for (j = 0; j < 10; j++) {
pthread_mutex_lock(&mutex);
while (count == 0) {
printf("consumer %d is waiting because buffer is empty\n", *id);
pthread_cond_wait(&empty, &mutex);
}
int data = buffer[out];
printf("consumer %d gets %d from buffer[%d]\n", *id, data, out);
out = (out + 1) % BUFFER_SIZE;
count--;
pthread_cond_signal(&full);
pthread_mutex_unlock(&mutex);
usleep(rand() % 1000);
}
}
pthread_exit(NULL);
}
int main()
{
pthread_t tid[6];
int id[6];
int i;
srand(time(NULL));
for (i = 0; i < 6; i++) {
id[i] = i + 1;
if (i % 2 == 0) {
pthread_create(&tid[i], NULL, producer, &id[i]);
} else {
pthread_create(&tid[i], NULL, consumer, &id[i]);
}
}
for (i = 0; i < 6; i++) {
pthread_join(tid[i], NULL);
}
return 0;
}
```
代码说明:
- 缓冲区用环形队列实现,使用三个变量 `in`、`out` 和 `count` 分别表示生产者写入数据的位置、消费者读取数据的位置和缓冲区中正在存储的数据个数。
- 使用互斥锁 `mutex` 对缓冲区进行互斥操作,确保每次只能有一个线程访问缓冲区。
- 生产者线程在生产数据时,如果缓冲区已满,则等待消费者线程取走数据后再进行生产,使用条件变量 `full` 实现等待和唤醒。
- 消费者线程在消费数据时,如果缓冲区为空,则等待生产者线程生产数据后再进行消费,使用条件变量 `empty` 实现等待和唤醒。
- 生产者和消费者分别循环操作 10 次。
- 最后使用 `pthread_join()` 函数等待所有线程执行结束。
相关推荐
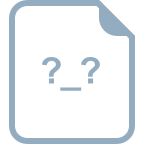
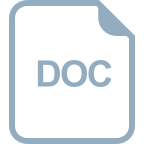
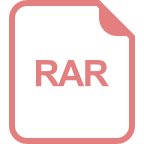
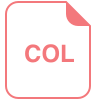
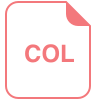
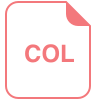
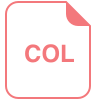
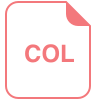









