用C语言实现生产者-消费者问题的模拟生产者消费者对缓冲区进行互斥操作。 缓冲区大小为10,缓冲区满则不允许生产者生产数据,缓冲区空则不允许消费者消费数据。 生产者消费者各循环操作10次。
时间: 2024-05-15 17:20:11 浏览: 13
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE]; // 缓冲区
int in = 0; // 缓冲区中下一个空位的索引
int out = 0; // 缓冲区中下一个数据的索引
int count = 0; // 缓冲区中当前存储的数据数量
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; // 互斥锁
pthread_cond_t empty = PTHREAD_COND_INITIALIZER; // 缓冲区空条件变量
pthread_cond_t full = PTHREAD_COND_INITIALIZER; // 缓冲区满条件变量
void *producer(void *arg) {
int i;
for (i = 0; i < 10; i++) {
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) { // 缓冲区满,等待消费者消费
printf("Buffer is full, producer is waiting...\n");
pthread_cond_wait(&empty, &mutex);
}
buffer[in] = rand() % 100; // 生产数据
printf("Producer produces %d, count = %d\n", buffer[in], count + 1);
in = (in + 1) % BUFFER_SIZE; // 更新下一个空位的索引
count++;
pthread_cond_signal(&full); // 唤醒等待缓冲区满条件变量的消费者
pthread_mutex_unlock(&mutex);
}
return NULL;
}
void *consumer(void *arg) {
int i;
for (i = 0; i < 10; i++) {
pthread_mutex_lock(&mutex);
while (count == 0) { // 缓冲区空,等待生产者生产
printf("Buffer is empty, consumer is waiting...\n");
pthread_cond_wait(&full, &mutex);
}
int data = buffer[out]; // 消费数据
printf("Consumer consumes %d, count = %d\n", data, count - 1);
out = (out + 1) % BUFFER_SIZE; // 更新下一个数据的索引
count--;
pthread_cond_signal(&empty); // 唤醒等待缓冲区空条件变量的生产者
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
pthread_t tid1, tid2;
srand(time(NULL));
pthread_create(&tid1, NULL, producer, NULL);
pthread_create(&tid2, NULL, consumer, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
```
相关推荐
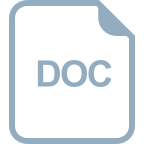














