c语言模拟实现生产者-消费者问题
时间: 2024-05-11 16:19:45 浏览: 8
生产者-消费者问题是一个经典的同步问题,其主要目的是协调多个线程之间的生产和消费行为,以避免数据竞争和死锁等问题。下面是一个基于 C 语言的生产者-消费者问题的模拟实现。
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10 // 缓冲区大小
#define PRODUCER_NUM 5 // 生产者数量
#define CONSUMER_NUM 5 // 消费者数量
int buffer[BUFFER_SIZE]; // 缓冲区数组
int count = 0; // 缓冲区中的元素数量
int in = 0; // 生产者将要插入的位置
int out = 0; // 消费者将要取出的位置
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t buffer_not_full; // 缓冲区不满的条件变量
pthread_cond_t buffer_not_empty; // 缓冲区不空的条件变量
void *producer(void *arg) // 生产者线程函数
{
int id = *(int *)arg;
free(arg);
while (1) {
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) { // 缓冲区已满
printf("producer %d is waiting because buffer is full.\n", id);
pthread_cond_wait(&buffer_not_full, &mutex); // 等待缓冲区不满
}
buffer[in] = 1; // 生产者生产一个物品
printf("producer %d produces a item at position %d.\n", id, in);
in = (in + 1) % BUFFER_SIZE; // 更新插入位置
count++; // 更新缓冲区元素数量
pthread_cond_signal(&buffer_not_empty); // 发出缓冲区不空的信号
pthread_mutex_unlock(&mutex);
}
}
void *consumer(void *arg) // 消费者线程函数
{
int id = *(int *)arg;
free(arg);
while (1) {
pthread_mutex_lock(&mutex);
while (count == 0) { // 缓冲区为空
printf("consumer %d is waiting because buffer is empty.\n", id);
pthread_cond_wait(&buffer_not_empty, &mutex); // 等待缓冲区不空
}
buffer[out] = 0; // 消费者消费一个物品
printf("consumer %d consumes a item at position %d.\n", id, out);
out = (out + 1) % BUFFER_SIZE; // 更新取出位置
count--; // 更新缓冲区元素数量
pthread_cond_signal(&buffer_not_full); // 发出缓冲区不满的信号
pthread_mutex_unlock(&mutex);
}
}
int main()
{
pthread_t tid;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&buffer_not_full, NULL);
pthread_cond_init(&buffer_not_empty, NULL);
for (int i = 0; i < PRODUCER_NUM; i++) {
int *arg = (int *)malloc(sizeof(int));
*arg = i;
pthread_create(&tid, NULL, producer, arg);
}
for (int i = 0; i < CONSUMER_NUM; i++) {
int *arg = (int *)malloc(sizeof(int));
*arg = i;
pthread_create(&tid, NULL, consumer, arg);
}
pthread_exit(NULL);
}
```
在这个模拟实现中,我们定义了一个大小为 BUFFER_SIZE 的缓冲区数组和三个线程同步对象:互斥锁 mutex,以及两个条件变量 buffer_not_full 和 buffer_not_empty。生产者线程通过循环不断地向缓冲区中插入物品,消费者线程通过循环不断地从缓冲区中取出物品,当缓冲区已满或为空时,线程会进入等待状态。当有物品被插入或取出时,线程会发出条件信号以唤醒其他线程。
在主函数中,我们创建了 PRODUCER_NUM 个生产者线程和 CONSUMER_NUM 个消费者线程,并通过调用 pthread_create 函数创建线程。最后,我们调用 pthread_exit 函数等待所有线程结束。
需要注意的是,这里的模拟实现中仅仅是为了演示生产者-消费者问题的基本思路和流程,实际应用中还需要考虑更多的细节问题,如线程的同步和互斥、缓冲区的容量大小、生产者和消费者的生产和消费速率等等。
相关推荐
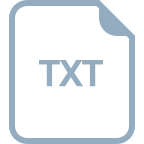
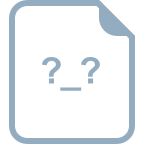













