python怎样绘制一个圆环图,使得圆环平均地被分为15个部分,每个部分的颜色不同,并且分别被标签为‘A’到'O,'写出代码
时间: 2023-03-10 12:13:57 浏览: 91
你可以使用 matplotlib 库绘制一个圆环图,代码如下:import matplotlib.pyplot as plt
import numpy as np labels = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O']
values = np.ones(15) fig, ax = plt.subplots()
ax.axis('equal')
ax.pie(values, labels=labels, radius=1, colors=plt.cm.rainbow(np.linspace(0, 1, 15)))
plt.show()
相关问题
python制如下图的4个同心圆环,其中红色圆环对应的半径为100像素,每个圆环宽度为25像素
在Python中,我们可以使用PIL(Python Imaging Library)库来创建这种效果,但现在更推荐使用其更新版Pillow。这里是一个简单的示例,展示如何使用Pillow来制作四个同心圆环:
```python
from PIL import Image, ImageDraw
# 创建一个新的白色背景图像
image_width = 600
image_height = 600
background_color = (255, 255, 255)
img = Image.new('RGB', (image_width, image_height), background_color)
# 创建画笔
draw = ImageDraw.Draw(img)
# 定义圆环的参数
rings = [(100, (255, 0, 0)), (75, (0, 255, 0)), (50, (0, 0, 255)), (25, (128, 0, 128))] # 半径和颜色
for radius, color in rings:
# 计算圆环的外边缘和内边缘的坐标
outer_radius = radius + 25
inner_radius = radius - 25
x, y = 0, 0 # 圆心默认在左上角
draw.ellipse((x, y, x+outer_radius, y+outer_radius), fill=color) # 绘制外部圆环
draw.ellipse((x+inner_radius, y+inner_radius, x+outer_radius, y+outer_radius), outline=color, width=2) # 绘制内部圆环
# 保存图片
img.save("concentric_circles.png")
```
这段代码会生成一个名为"concentric_circles.png"的图片文件,它包含四个同心圆环,最外面的是红色的,然后依次是绿色、蓝色和紫色。
绘制如下图的4个同心圆环,其中红色圆环对应的半径为100像素,每个圆环宽度为25像素
要绘制四个同心圆环,你可以使用各种图形库,比如在HTML5的Canvas上,或者使用像Python的matplotlib这样的绘图工具。这里我会给出一个简单的示例说明如何用Python和matplotlib来实现这个效果:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建一个新的figure
fig, ax = plt.subplots()
# 设置背景颜色为白色
ax.set_facecolor('white')
# 定义圆环的颜色和半径
rings = [100, 75, 50, 25] # 半径列表
colors = ['red', 'green', 'blue', 'yellow'] # 颜色列表
# 对于每个圆环,创建并填充一个圆形区域
for i in range(len(rings)):
outer_radius = rings[i]
inner_radius = rings[i] - 25 # 圆环宽度为25像素
circle = plt.Circle((0, 0), outer_radius, color=colors[i])
patch = plt.PathPatch(circle)
ax.add_patch(patch)
# 设置坐标轴范围,使其只显示圆环部分
ax.set_xlim(-100, 100)
ax.set_ylim(-100, 100)
# 显示图形
plt.show()
```
如果你想要更具体的步骤,例如在Web前端环境中,可以使用JavaScript的`<canvas>`标签结合HTML和CSS来实现。这是一个基本的HTML/CSS/JavaScript例子:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<style>
canvas {
background-color: white;
}
</style>
</head>
<body>
<canvas id="myCanvas" width="400" height="400"></canvas>
<script>
const ctx = document.getElementById('myCanvas').getContext('2d');
let radiusStep = 100;
for (let i = 0; i < 4; i++) {
drawCircle(ctx, i * radiusStep + 25, radiusStep);
radiusStep -= 25;
}
function drawCircle(ctx, x, r) {
ctx.beginPath();
ctx.arc(x, y, r, 0, Math.PI * 2);
ctx.fillStyle = `#${i % 360 * 3.6}; // 颜色对应0-360的HSV值,这里简化了颜色选择`
ctx.fill();
ctx.closePath();
}
</script>
</body>
</html>
```
阅读全文
相关推荐
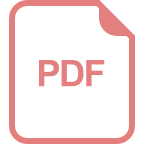
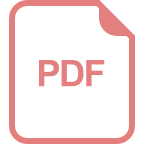
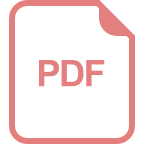
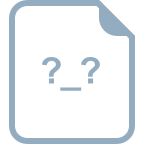
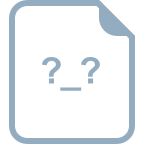
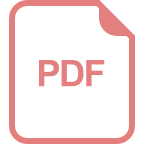
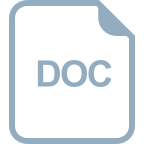




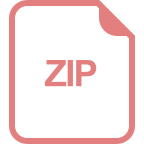