用python写一个连接SQLSERVER的代码,且有GUI界面
时间: 2023-09-06 19:04:59 浏览: 273
### 回答1:
可以使用 Python 的 pyodbc 库来连接和操作 SQL Server 数据库。下面是一个示例代码,用于连接并执行一条查询:
```python
import pyodbc
# 连接字符串
conn_str = (
r'DRIVER={ODBC Driver 17 for SQL Server};'
r'SERVER=server_name;'
r'DATABASE=database_name;'
r'UID=username;'
r'PWD=password'
)
# 连接数据库
cnxn = pyodbc.connect(conn_str)
# 创建游标
cursor = cnxn.cursor()
# 执行查询
cursor.execute('SELECT * FROM table_name')
# 获取查询结果
result = cursor.fetchall()
# 处理结果
for row in result:
print(row)
# 关闭游标和连接
cursor.close()
cnxn.close()
```
如果想要在 Python 中使用 GUI,可以使用 Tkinter 模块。下面是一个示例代码,用于创建一个简单的 GUI 窗口:
```python
import tkinter as tk
# 创建主窗口
root = tk.Tk()
root.title("连接 SQL Server 示例")
# 创建文本输入框
server_name_entry = tk.Entry(root)
server_name_entry.pack()
# 创建按钮
def connect_to_server():
server_name = server_name_entry.get()
print("连接到服务器:", server_name)
connect_button = tk.Button(root, text="连接", command=connect_to_server)
connect_button.pack()
# 运行主循环
root.mainloop()
```
可以在 GUI 窗口中添加更多的组件,如文本标签、输入框、按钮等,来实现更复杂的功能。
### 回答2:
要在Python中连接SQL Server并创建一个带有GUI界面的代码,可以使用Python的Tkinter库来创建GUI界面,并使用pyodbc库来连接SQL Server数据库。以下是一个简单的示例代码:
```python
import tkinter as tk
import pyodbc
# 连接SQL Server数据库
conn = pyodbc.connect(
'DRIVER={SQL Server};SERVER=服务器名称;DATABASE=数据库名称;UID=用户名;PWD=密码')
# 创建GUI界面
root = tk.Tk()
root.title("SQL Server连接测试")
root.geometry("300x200")
# SQL查询函数
def execute_sql():
sql_query = entry.get() # 获取用户输入的SQL查询语句
cursor = conn.cursor()
cursor.execute(sql_query) # 执行SQL查询
results = cursor.fetchall() # 获取查询结果
cursor.close()
# 显示查询结果
result_text.delete(1.0, tk.END)
for row in results:
result_text.insert(tk.END, str(row) + "\n")
# 创建GUI组件
entry = tk.Entry(root, width=40)
entry.pack(pady=10)
execute_button = tk.Button(root, text="执行SQL查询", command=execute_sql)
execute_button.pack(pady=5)
result_text = tk.Text(root, height=8, width=40)
result_text.pack()
root.mainloop()
```
在上述代码中,需要根据你的实际情况修改连接SQL Server数据库的参数(服务器名称、数据库名称、用户名和密码)。代码创建了一个GUI界面,包括一个文本框用于输入SQL查询语句,一个按钮用于执行查询,以及一个文本框用于显示查询结果。用户输入的SQL查询语句将通过pyodbc库连接到SQL Server数据库,并将结果显示在界面上。
### 回答3:
在Python中连接SQL Server并创建一个有 GUI 界面的应用程序是可行的。下面是一个简单的示例代码:
```python
import tkinter as tk
import pyodbc
# 连接到 SQL Server 数据库
connection = pyodbc.connect('Driver={SQL Server};'
'Server=服务器名;'
'Database=数据库名;'
'UID=用户名;'
'PWD=密码;')
# 创建GUI窗口
window = tk.Tk()
window.title("SQL Server 连接测试")
window.geometry("300x200")
# 查询按钮点击事件处理函数
def query_data():
# 获取输入的SQL语句
sql = sql_entry.get()
# 执行SQL查询
cursor = connection.cursor()
cursor.execute(sql)
# 获取查询结果
result = cursor.fetchall()
# 显示结果
result_text.configure(state='normal')
result_text.delete('1.0', tk.END)
result_text.insert(tk.END, result)
result_text.configure(state='disabled')
# 输入框和按钮
sql_label = tk.Label(window, text="SQL语句:")
sql_label.pack()
sql_entry = tk.Entry(window, width=30)
sql_entry.pack()
query_button = tk.Button(window, text="查询", command=query_data)
query_button.pack()
# 结果显示区域
result_text = tk.Text(window, state='disabled')
result_text.pack()
window.mainloop()
# 关闭数据库连接
connection.close()
```
上述代码使用 `pyodbc` 第三方库来连接 SQL Server 数据库。在连接时,需要根据实际情况填写正确的服务器名、数据库名、用户名和密码。该示例创建了一个简单的 GUI 窗口,包括一个输入框用于输入 SQL 语句,一个查询按钮用于执行查询,以及一个文本框用于显示查询结果。
请注意:在实际使用时,需要根据具体的需求和环境,对以上代码进行相应的修改和优化。
阅读全文
相关推荐
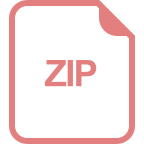
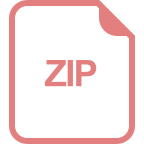


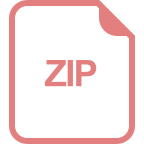
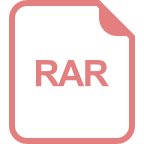
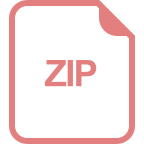
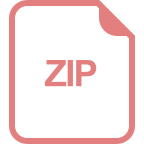
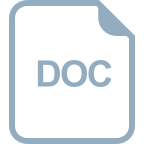
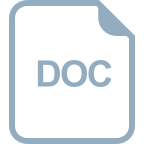
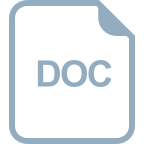






