帮我写一个C语言函数,判断该日期是否为周末
时间: 2023-09-16 08:05:24 浏览: 278
### 回答1:
好的,那么这是一个可以用来判断某个日期是否为周末的 C 语言函数:
```
#include <time.h>
int is_weekend(int year, int month, int day) {
// 将日期转换为 time_t 类型
struct tm tm = {0};
tm.tm_year = year - 1900;
tm.tm_mon = month - 1;
tm.tm_mday = day;
time_t time = mktime(&tm);
// 获取当前日期的星期
struct tm *local = localtime(&time);
int wday = local->tm_wday;
// 判断是否为周末
if (wday == 0 || wday == 6) {
return 1;
} else {
return 0;
}
}
```
使用方法:调用函数 `is_weekend(year, month, day)`,其中 year 为年份、month 为月份、day 为日。函数返回值为 1 时表示该日期为周末,返回值为 0 时表示不是周末。
例如,判断 2022 年 1 月 1 日是否为周末,可以这样调用函数:
```
int result = is_weekend(2022, 1, 1);
if (result == 1) {
printf("是周末\n");
} else {
printf("不是周末\n");
}
```
希望这个函数能够帮助到你。
### 回答2:
可以使用以下的C语言函数来判断给定的日期是否为周末:
```c
#include <stdio.h>
// 判断给定日期是否为周末
int isWeekend(int year, int month, int day) {
// 对于给定日期,我们需要先判断是否为周日
// 其余的周末日期都会比周日提前1或2天
// 所以只要判断是不是周日即可
// 公式 Zeller's Congruence
// 改编自 https://en.wikipedia.org/wiki/Zeller%27s_congruence
int h, q, m, k, j;
q = day;
m = month;
if (m < 3) {
m += 12;
year--;
}
k = year % 100;
j = year / 100;
h = (q + 13*(m+1)/5 + k + k/4 + j/4 + 5*j) % 7;
// 0表示周六, 1表示周日
if (h == 0 || h == 1) {
return 1;
} else {
return 0;
}
}
int main() {
int year, month, day;
printf("请输入日期(YYYY MM DD):");
scanf("%d%d%d", &year, &month, &day);
if (isWeekend(year, month, day)) {
printf("给定日期为周末");
} else {
printf("给定日期不是周末");
}
return 0;
}
```
这个函数使用了[Zeller's Congruence](https://en.wikipedia.org/wiki/Zeller%27s_congruence)的公式来计算给定日期的星期几,进而判断它是否为周末。在主函数中,我们通过用户输入获取要判断的日期,然后调用`isWeekend()`函数进行判断,最后输出结果。
### 回答3:
以下是一个用C语言编写的函数,用于判断给定的日期是否是周末:
```c
#include <stdio.h>
#include <stdbool.h>
bool isWeekend(int year, int month, int day)
{
// 根据日期计算出该天是星期几
int weekDay = 0; // 0表示星期日,1表示星期一,依此类推
int centuryCode = (year - 1) / 100 + 1; // 世纪码
int yearCode = (year - 1) % 100; // 年码
weekDay = (centuryCode / 4 - 2 * centuryCode + yearCode + yearCode / 4 + 13 * (month + 1) / 5 + day - 1) % 7;
// 判断计算出的星期几是否是周末
if (weekDay == 0 || weekDay == 6) {
return true; // 星期日或星期六为周末
} else {
return false; // 其他工作日
}
}
int main()
{
int year, month, day;
printf("请输入日期(以YYYY MM DD的格式输入):");
scanf("%d %d %d", &year, &month, &day);
bool result = isWeekend(year, month, day);
if (result) {
printf("该日期是周末\n");
} else {
printf("该日期是工作日\n");
}
return 0;
}
```
阅读全文
相关推荐

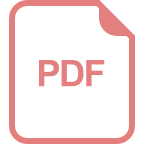
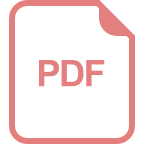
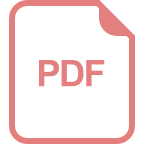
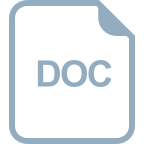
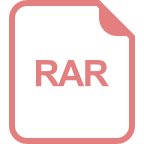
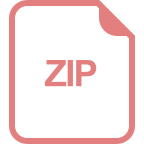
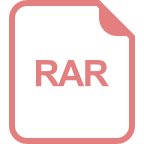
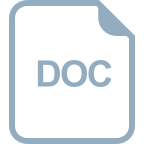




