使用python代码举例说明旅行商问题
时间: 2023-09-19 17:06:41 浏览: 102
### 回答1:
旅行商问题是指,有一个旅行者要去访问若干个城市,在这些城市之间旅行时会产生一定的代价,问题是要求出旅行者在访问这些城市的最小代价。
例如,给定一张有向图,其中有四个城市A、B、C、D,旅行者想要访问这四个城市。访问每两个城市之间的代价如下所示:
A -> B: 10
A -> C: 20
A -> D: 15
B -> C: 10
B -> D: 35
C -> D: 30
那么,旅行者可以选择从A出发,先走到B,再走到C,最后走到D,总代价为10+10+30=50。或者从A出发,直接走到D,总代价为15。因此,旅行者在访问这四个城市的最小代价是15。
下面是使用Python代码来求解旅行商问题的示例:
```python
from itertools import permutations
# 城市数量
num_cities = 4
# 访问每两个城市之间的代价
costs = [[0, 10, 20, 15],
[0, 0, 10, 35],
[0, 0, 0, 30],
[0, 0, 0, 0]]
# 计算从一个城市出发,访问所有城市的最小代价
def calc_min_cost(start):
# 得到所有城市的排列
perms = permutations(range(1, num_cities))
# 记录最小代价
min_cost = float('inf')
# 遍历所有排列
for perm in perms:
# 记录当前代价
cost = 0
# 从
### 回答2:
旅行商问题(TSP)是一个著名的组合优化问题,其目标是找到一个旅行商从一个城市出发,经过指定的一系列城市后再回到出发城市的最短路径,同时保证每个城市只经过一次。
以下是使用Python代码实现TSP问题的一个简单例子:
```python
import numpy as np
from itertools import permutations
# 构建城市之间的距离矩阵
distances = np.array([[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]])
# 计算旅行商问题的最短路径
def tsp(distances):
num_cities = distances.shape[0] # 城市数量
shortest_path = None # 最短路径
shortest_distance = float('inf') # 最短路径的长度
# 生成所有可能的路径
for path in permutations(range(1, num_cities)):
current_distance = 0 # 当前路径的长度
previous_city = 0 # 上一个访问的城市
# 计算当前路径的长度
for city in path:
current_distance += distances[previous_city, city]
previous_city = city
# 加上返回原始城市的距离
current_distance += distances[previous_city, 0]
# 更新最短路径和长度
if current_distance < shortest_distance:
shortest_distance = current_distance
shortest_path = path
return shortest_path, shortest_distance
# 打印最短路径和长度
shortest_path, shortest_distance = tsp(distances)
print("最短路径:", shortest_path)
print("最短路径长度:", shortest_distance)
```
在上述代码中,我们首先定义了一个距离矩阵,该矩阵表示了城市之间的距离。然后,我们定义了一个名为`tsp`的函数,该函数使用了`permutations`函数来生成所有可能的路径,并使用一个循环来计算每条路径的长度。在计算完所有路径的长度后,我们根据长度来更新最短路径和最短路径长度。最后,我们调用`tsp`函数,并打印出最短路径和最短路径长度。
这个例子只是TSP问题的一个简单示例,实际上TSP问题很复杂,特别是在城市数量较大时。为了提高效率,可以使用一些优化技术,例如动态规划和启发式算法,来减少计算时间。
### 回答3:
旅行商问题(Traveling Salesman Problem,TSP)是一种经典的优化问题,其目标是找到一条最短的路径,使得旅行商能够只经过每个城市一次,并最终回到起始城市。
下面是使用Python代码举例说明旅行商问题的思路:
```python
import itertools
# 定义城市集合和距离矩阵
cities = ["A", "B", "C", "D"]
distances = {
("A", "B"): 10,
("A", "C"): 15,
("A", "D"): 20,
("B", "C"): 35,
("B", "D"): 25,
("C", "D"): 30
}
# 定义计算路径长度的函数
def calculate_distance(path):
total_distance = 0
for i in range(len(path)-1):
total_distance += distances[(path[i], path[i+1])]
return total_distance
# 生成所有可能的路径
all_paths = list(itertools.permutations(cities))
# 初始化最短路径和最短距离
shortest_path = None
shortest_distance = float('inf')
# 遍历所有路径,计算距离并更新最短路径和最短距离
for path in all_paths:
distance = calculate_distance(path)
if distance < shortest_distance:
shortest_distance = distance
shortest_path = path
# 输出最短路径和最短距离
print("最短路径为:", shortest_path)
print("最短距离为:", shortest_distance)
```
上述代码首先定义了一个城市集合和城市之间的距离矩阵。然后使用`itertools.permutations()`函数生成了所有可能的路径。接下来,定义了一个函数`calculate_distance()`用于计算路径长度。代码使用一个循环遍历所有路径,并通过调用`calculate_distance()`函数计算每个路径的长度。最后,取得最短路径和最短距离,并输出结果。
这段代码可以根据不同的城市和距离矩阵进行修改和扩展,以解决更复杂的旅行商问题。
阅读全文
相关推荐














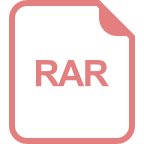