Python 完整代码示例,SEIR模型,直接写代码
时间: 2023-09-09 13:04:22 浏览: 218
### 回答1:
SEIR模型是一种常用的传染病模型,用于模拟传染病在人群之间的传播。SEIR模型包含四个状态:
- S(易感):健康,未感染传染病的人。
- E(潜伏):已感染传染病,但还未发病的人。
- I(感染):已感染传染病,并且已经发病的人。
- R(恢复):已恢复的人。
下面是一个使用Python实现SEIR模型的完整代码示例:
```python
import numpy as np
from scipy.integrate import odeint
import matplotlib.pyplot as plt
# 设置参数
beta = 0.3 # 传播系数
gamma = 0.1 # 恢复率
sigma = 1.0 / 3.0 # 潜伏期持续时间
N = 1000 # 总人数
# 定义SEIR模型
def seir_model(y, t, beta, gamma, sigma):
S, E, I, R = y
dSdt = -beta * S * I / N
dEdt = beta * S * I / N - sigma * E
dIdt = sigma * E - gamma * I
dRdt = gamma * I
return dSdt, dEdt, dIdt, dRdt
# 初始化参数
S0, E0, I0, R0 = 999, 1, 0, 0 # 假设有1个人已感染,其余999个人健康
y0 = [S0, E0, I0, R0]
# 设置时间范围
t = np.linspace(0, 100, 100)
# 解决方程
result = odeint(seir_model, y0, t, args=(beta, gamma, sigma))
S, E, I, R = result.T
# 绘制曲线图
plt.plot(t, S, label='Susceptible')
plt.plot(t, E, label='Exposed')
plt
### 回答2:
以下是一个使用Python编写的SEIR模型的完整代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
def seir_model(beta, gamma, sigma, initial_population, initial_exposed, initial_infected, initial_recovered, days):
population = np.zeros(days)
exposed = np.zeros(days)
infected = np.zeros(days)
recovered = np.zeros(days)
population[0] = initial_population
exposed[0] = initial_exposed
infected[0] = initial_infected
recovered[0] = initial_recovered
for t in range(1, days):
dP = -beta * exposed[t-1] * infected[t-1] / population[t-1]
dE = beta * exposed[t-1] * infected[t-1] / population[t-1] - sigma * exposed[t-1]
dI = sigma * exposed[t-1] - gamma * infected[t-1]
dR = gamma * infected[t-1]
population[t] = population[t-1] + dP
exposed[t] = exposed[t-1] + dE
infected[t] = infected[t-1] + dI
recovered[t] = recovered[t-1] + dR
return population, exposed, infected, recovered
if __name__ == "__main__":
beta = 0.5
gamma = 0.2
sigma = 0.1
initial_population = 1000
initial_exposed = 10
initial_infected = 1
initial_recovered = 0
days = 100
population, exposed, infected, recovered = seir_model(beta, gamma, sigma, initial_population, initial_exposed, initial_infected, initial_recovered, days)
plt.plot(population, label='Population')
plt.plot(exposed, label='Exposed')
plt.plot(infected, label='Infected')
plt.plot(recovered, label='Recovered')
plt.xlabel('Days')
plt.ylabel('Number of Individuals')
plt.title('SEIR Model')
plt.legend()
plt.show()
```
代码中的`seir_model`函数定义了一个SEIR模型,根据给定的参数计算出每天的人群数量,并将结果以四条曲线的形式绘制出来。在`if __name__ == "__main__"`中,我们定义了一些初始参数,并调用`seir_model`函数进行模拟,并将结果绘制成曲线图展示出来。
相关推荐
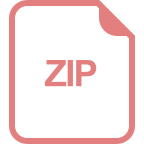










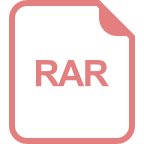