用Pytorch写SDNE代码。
时间: 2023-09-06 08:05:04 浏览: 80
### 回答1:
好的,我来为您解释一下如何用 PyTorch 实现 SDNE 算法。
首先,您需要准备好一个网络的邻接矩阵和一些超参数,例如隐藏层大小、学习率等。
然后,您可以使用 PyTorch 的 `nn.Module` 类来定义一个 SDNE 网络模型,其中包含两个全连接层和一个自编码器。
接着,您可以定义损失函数和优化器,并使用 PyTorch 的自动求导功能来计算损失函数的梯度。
最后,您可以使用 PyTorch 的训练循环来训练模型,并在训练过程中不断更新权重。
下面是一个简单的例子,展示了如何使用 PyTorch 实现 SDNE 算法的基本流程:
```python
import torch
import torch.nn as nn
# 定义超参数
input_size = 10
hidden_size = 20
num_classes = 5
learning_rate = 0.001
# 定义网络
class SDNE(nn.Module):
def __init__(self, input_size, hidden_size, num_classes):
super(SDNE, self).__init__()
self.fc1 = nn.Linear(input_size, hidden_size)
self.fc2 = nn.Linear(hidden_size, num_classes)
self.encoder = nn.Sequential(
nn.Linear(input_size, hidden_size),
nn.ReLU(),
nn.Linear(hidden_size, hidden_size),
nn.ReLU()
)
def forward(self, x):
out = self.fc1(x)
out = self.fc2(out)
out = self.encoder(out)
return out
# 实例化模型
model = SD
### 回答2:
SDNE(Structural Deep Network Embedding)是一种用于图形嵌入的深度学习方法,可以将图形中的节点映射到低维空间以捕获其结构信息。以下是使用PyTorch编写SDNE代码的简要步骤:
1. 导入PyTorch库和其他所需库。
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
```
2. 定义SDNE模型类,继承自nn.Module。
```python
class SDNE(nn.Module):
def __init__(self, input_dim, hidden_dim, embedding_dim):
super(SDNE, self).__init__()
self.encoder = nn.Sequential(
nn.Linear(input_dim, hidden_dim),
nn.ReLU(),
nn.Linear(hidden_dim, embedding_dim)
)
def forward(self, x):
encoded = self.encoder(x)
return encoded
```
3. 定义训练函数,包括数据预处理、模型实例化、损失函数和优化器的定义,以及训练迭代。
```python
def train(model, data, num_epochs, learning_rate):
# 数据预处理
x = torch.from_numpy(data).float()
# 模型实例化
model = SDNE(x.shape[1], hidden_dim, embedding_dim)
# 损失函数和优化器定义
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
# 训练迭代
for epoch in range(num_epochs):
optimizer.zero_grad()
encoded = model(x)
loss = criterion(encoded, x)
loss.backward()
optimizer.step()
if (epoch+1) % 10 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
```
4. 调用训练函数进行训练。
```python
data = np.random.randn(100, 10) # 示例数据
num_epochs = 100
learning_rate = 0.001
hidden_dim = 50
embedding_dim = 20
model = SDNE(data.shape[1], hidden_dim, embedding_dim)
train(model, data, num_epochs, learning_rate)
```
以上是一个简单的使用PyTorch编写SDNE代码的示例,实际应用中可能需要根据具体情况进行调整和扩展。
相关推荐
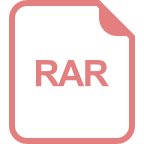










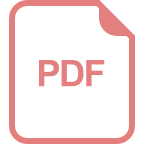