pytorch实现SDNE代码
时间: 2023-04-07 15:00:35 浏览: 54
SDNE(Stacked Denoising Autoencoder)是一种用于网络嵌入的有效方法。下面是使用 PyTorch 实现 SDNE 的示例代码:
```python
import torch
import torch.nn as nn
class SDNE(nn.Module):
def __init__(self, input_dim, hidden_dims, output_dim, dropout=0.5):
super(SDNE, self).__init__()
self.input_dim = input_dim
self.output_dim = output_dim
self.hidden_dims = hidden_dims
self.dropout = dropout
self.encoder_layers = nn.ModuleList()
self.decoder_layers = nn.ModuleList()
# Add encoder layers
in_dim = self.input_dim
for i, h_dim in enumerate(self.hidden_dims):
self.encoder_layers.append(nn.Linear(in_dim, h_dim))
self.encoder_layers.append(nn.ReLU())
self.encoder_layers.append(nn.Dropout(p=self.dropout))
in_dim = h_dim
# Add final encoder layer
self.encoder_layers.append(nn.Linear(in_dim, self.output_dim))
# Add decoder layers
in_dim = self.output_dim
for i, h_dim in enumerate(reversed(self.hidden_dims)):
self.decoder_layers.append(nn.Linear(in_dim, h_dim))
self.decoder_layers.append(nn.ReLU())
self.decoder_layers.append(nn.Dropout(p=self.dropout))
in_dim = h_dim
# Add final decoder layer
self.decoder_layers.append(nn.Linear(in_dim, self.input_dim))
def forward(self, x):
# Encode input
encoded = x
for layer in self.encoder_layers:
encoded = layer(encoded)
# Decode encoded input
decoded = encoded
for layer in self.decoder_layers:
decoded = layer(decoded)
return encoded, decoded
```
在这段代码中,我们定义了一个 PyTorch 模型 `SDNE`,它继承自 `nn.Module` 类。我们在构造函数中定义了输入维度、隐藏层维度列表、输出维度和 dropout 的概率。
相关推荐









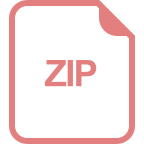
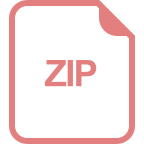