springboot整合flowable教程
时间: 2023-05-31 21:18:30 浏览: 171
### 回答1:
Spring Boot整合Flowable的教程可以分为以下几个步骤:
1. 引入依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.flowable</groupId>
<artifactId>flowable-spring-boot-starter</artifactId>
<version>6.6.</version>
</dependency>
```
2. 配置数据源
在application.properties文件中配置数据源:
```
spring.datasource.url=jdbc:mysql://localhost:3306/flowable?useUnicode=true&characterEncoding=utf8&useSSL=false
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
3. 配置Flowable
在application.properties文件中配置Flowable:
```
# 配置Flowable的数据库表前缀
spring.flowable.database-schema-update=true
spring.flowable.database-schema=flowable
spring.flowable.database-schema-update=true
```
4. 编写流程定义
在resources目录下创建一个名为processes的文件夹,用于存放流程定义的bpmn文件。例如,创建一个名为leave.bpmn的文件,内容如下:
```
<?xml version="1." encoding="UTF-8"?>
<definitions xmlns="http://www.omg.org/spec/BPMN/20100524/MODEL"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:flowable="http://flowable.org/bpmn"
targetNamespace="http://activiti.org/bpmn20"
xsi:schemaLocation="http://www.omg.org/spec/BPMN/20100524/MODEL BPMN20.xsd"
expressionLanguage="http://www.w3.org/1999/XPath"
typeLanguage="http://www.w3.org/2001/XMLSchema">
<process id="leave" name="请假流程">
<startEvent id="start" name="开始"/>
<userTask id="apply" name="申请请假"/>
<sequenceFlow id="flow1" sourceRef="start" targetRef="apply"/>
<endEvent id="end" name="结束"/>
<sequenceFlow id="flow2" sourceRef="apply" targetRef="end"/>
</process>
</definitions>
```
5. 编写流程服务
创建一个名为ProcessService的服务类,用于启动流程实例:
```
@Service
public class ProcessService {
@Autowired
private RuntimeService runtimeService;
public void startProcess(String processDefinitionKey) {
runtimeService.startProcessInstanceByKey(processDefinitionKey);
}
}
```
6. 编写控制器
创建一个名为ProcessController的控制器类,用于处理请求:
```
@RestController
public class ProcessController {
@Autowired
private ProcessService processService;
@PostMapping("/startProcess")
public void startProcess() {
processService.startProcess("leave");
}
}
```
7. 启动应用程序
运行Spring Boot应用程序,访问http://localhost:808/startProcess即可启动流程实例。
以上就是Spring Boot整合Flowable的教程,希望对你有所帮助。
### 回答2:
SpringBoot是Spring框架的一款快速开发框架,而Flowable则是一款基于Activiti的轻量级工作流引擎。SpringBoot整合Flowable可以快速开发出支持业务流程的应用程序。
1. 创建SpringBoot项目
首先,我们需要创建一个SpringBoot项目。可以使用Spring官网提供的Spring Initializr快速创建。
2. 添加Flowable依赖
在pom.xml文件中添加Flowable的依赖。
```
<dependency>
<groupId>org.flowable</groupId>
<artifactId>flowable-spring-boot-starter</artifactId>
<version>6.3.1</version>
</dependency>
```
3. 配置Flowable
在application.yml配置文件中添加Flowable相关配置。
```
flowable:
database-schema-update: true
history-level: full
id-generator: uuid
jdbc-url: jdbc:mysql://localhost:3306/flowable-spring-boot?useUnicode=true&characterEncoding=utf-8&useSSL=false
jdbc-username: root
jdbc-password: root
rest:
enabled: true
base-path: /flowable-rest
```
其中,jdbc-url为数据库连接地址,jdbc-username和jdbc-password为连接数据库的用户名和密码。
4. 创建业务流程定义
在src/main/resources目录下创建bpmn和png文件,用于定义业务流程。
5. 配置业务流程服务
在Java代码中配置业务流程服务。
```
@Configuration
public class FlowableConfiguration {
@Autowired
private DataSource dataSource;
@Bean
public StandaloneProcessEngineConfiguration processEngineConfiguration() {
StandaloneProcessEngineConfiguration configuration = new StandaloneProcessEngineConfiguration();
configuration.setDataSource(dataSource);
configuration.setDatabaseSchemaUpdate(ProcessEngineConfiguration.DB_SCHEMA_UPDATE_TRUE);
configuration.setAsyncExecutorActivate(true);
configuration.setHistory(ProcessEngineConfiguration.HISTORY_AUDIT);
return configuration;
}
@Bean
public ProcessEngine processEngine() {
return processEngineConfiguration().buildProcessEngine();
}
@Bean
public RepositoryService repositoryService() {
return processEngine().getRepositoryService();
}
@Bean
public RuntimeService runtimeService() {
return processEngine().getRuntimeService();
}
@Bean
public TaskService taskService() {
return processEngine().getTaskService();
}
@Bean
public HistoryService historyService() {
return processEngine().getHistoryService();
}
}
```
6. 创建业务流程控制器
创建一个控制器类,用于处理业务流程的相关操作。
```
@RestController
@RequestMapping("/processes")
public class ProcessController {
@Autowired
private RuntimeService runtimeService;
@Autowired
private TaskService taskService;
@PostMapping("/start")
public void startProcessInstance() {
runtimeService.startProcessInstanceByKey("myProcess");
}
@GetMapping("/tasks/{assignee}")
public List<Task> getTasks(@PathVariable("assignee") String assignee) {
return taskService.createTaskQuery().taskAssignee(assignee).list();
}
@PostMapping("/complete/{taskId}")
public void completeTask(@PathVariable("taskId") String taskId) {
taskService.complete(taskId);
}
}
```
7. 启动应用程序
最后,我们可以启动应用程序,在浏览器中访问 http://localhost:8080/flowable-rest/runtime/process-instances 可以查看当前正在运行的业务流程实例。
通过上述步骤,我们可以实现SpringBoot整合Flowable的功能,并快速开发出支持业务流程的应用程序。
### 回答3:
Spring Boot是现今非常流行的Java开发框架,而Flowable则是一个非常强大的开源工作流引擎。将二者进行整合可以使工作流的实现更加简单高效,能够帮助开发人员快速构建出完整的业务系统。
以下是Spring Boot整合Flowable的具体步骤:
1. 加入Flowable包依赖
在项目的pom.xml文件中加入以下依赖代码即可引入Flowable:
```
<dependency>
<groupId>org.flowable</groupId>
<artifactId>flowable-spring-boot-starter</artifactId>
<version>${flowable.version}</version>
</dependency>
```
其中,${flowable.version}需要根据所使用版本进行修改。
2. Flowable配置文件
在src/main/resources目录下新建flowable.cfg.xml文件。该文件中定义了Flowable框架的数据库配置信息。
示例代码:
```
<?xml version="1.0" encoding="UTF-8"?>
<flowable xmlns="http://flowable.org/schema/flowable">
<datasource>
<url>jdbc:mysql://localhost:3306/db_flowable?autoReconnect=true&useUnicode=true&characterEncoding=utf-8&serverTimezone=Asia/Shanghai</url>
<driver>com.mysql.cj.jdbc.Driver</driver>
<username>root</username>
<password>123456</password>
<maxActive>20</maxActive>
<maxIdle>2</maxIdle>
<maxWait>10000</maxWait>
</datasource>
<job-executor activate="true" />
<process-engine>
<name>default</name>
<database-schema-update>true</database-schema-update>
<history-level>full</history-level>
<configuration-resource>flowable.properties</configuration-resource>
</process-engine>
</flowable>
```
其中,此处示例使用的是MySQL数据库,如果要使用其他类型的数据库需要进行对应修改。
3. Flowable属性文件
在src/main/resources目录下新建flowable.properties文件。该文件中定义了Flowable框架的各种配置信息,如邮件服务器、任务分配策略等。
示例代码:
```
# 邮件服务器配置
mail.server.host=smtp.163.com
mail.server.port=465
mail.server.username=your@mail.com
mail.server.password=xxx
# 任务分配策略
activiti.bpmn.userTask.assignmentStrategy=org.flowable.spring.boot.app.task.SimpleTaskAssignmentStrategy
```
其中,示例代码中的邮件服务器为163邮箱服务器,如果使用其他邮箱需要进行对应修改。任务分配策略也可根据具体需求进行修改。
4. 编写工作流程定义文件
在src/main/resources/processes目录下新建flowable配置文件,使用BPMN2.0的XML格式描述组织的流程图。
示例代码:
```
<?xml version="1.0" encoding="UTF-8"?>
<definitions xmlns="http://www.omg.org/spec/BPMN/20100524/MODEL"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:activiti="http://activiti.org/bpmn"
targetNamespace="http://www.activiti.org/bpmn"
xsi:schemaLocation="http://www.omg.org/spec/BPMN/20100524/MODEL BPMN20.xsd http://activiti.org/bpmn http://activiti.org/bpmn">
<process id="myProcess" name="My process">
<startEvent id="start" activiti:formKey="key_start"></startEvent>
<sequenceFlow id="flow1" sourceRef="start" targetRef="task1"></sequenceFlow>
<userTask id="task1" name="任务1" activiti:assignee="userId1" activiti:formKey="key_task1"></userTask>
<sequenceFlow id="flow2" sourceRef="task1" targetRef="end"></sequenceFlow>
<endEvent id="end"></endEvent>
</process>
</definitions>
```
其中,activiti:formKey为工作流程表单路径,activiti:assignee为任务的受让人。
5. 编写Java代码
- 启动方式
在启动类中加上@EnableFlowable注解即可启动Flowable引擎。
```
@SpringBootApplication
@EnableFlowable
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
- 工作流程定义
在Java类中使用以下代码即可进行工作流程的定义:
```
@RepositoryRestController
@RequestMapping("/workflow-definition")
public class WorkflowDefinitionController {
@Autowired
private RepositoryService repositoryService;
@PostMapping("/deploy")
public void deploy(@RequestParam("file") MultipartFile file) throws Exception {
if (file.isEmpty()) {
throw new IllegalArgumentException("文件不能为空");
}
Deployment deployment = repositoryService.createDeployment()
.addInputStream(file.getOriginalFilename(), file.getInputStream())
.deploy();
}
}
```
在示例代码中,@RepositoryRestController注解用于定义RESTful接口,@RequestMapping注解用于映射URI路径。
- 工作流程实例
使用以下代码即可开启一个工作流程实例:
```
@RestController
@RequestMapping("/process")
public class ProcessController {
@Autowired
private RuntimeService runtimeService;
@PostMapping("/start")
public void start(@RequestParam String processDefinitionKey) {
runtimeService.startProcessInstanceByKey(processDefinitionKey);
}
}
```
6. 运行工作流
启动Spring Boot程序,并访问RESTful接口所映射的URL,即可实现工作流的运行。
以上就是Spring Boot整合Flowable的教程,通过以上步骤,可以非常快速地搭建出一个高效的工作流系统,帮助开发人员实现更加快速、可靠的业务流程。
阅读全文
相关推荐
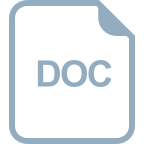
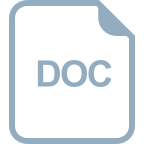
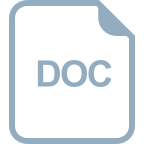
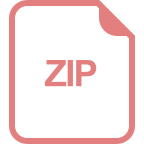





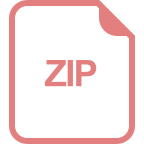
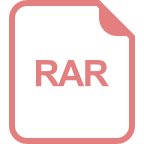
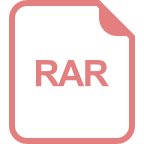
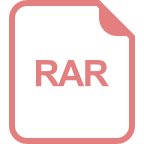
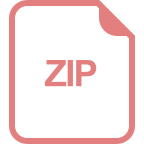
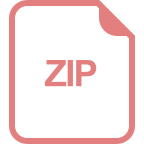
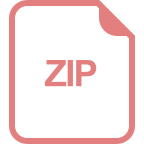
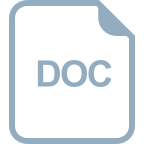
