java实现拷贝当前工作空间所有文件(含目录)
时间: 2023-09-25 22:06:36 浏览: 125
### 回答1:
可以使用 Java 的 `File` 类和 `FileInputStream` 以及 `FileOutputStream` 类来实现拷贝文件的功能。下面是一个示例代码:
```
import java.io.*;
public class CopyDirectory {
public static void main(String[] args) {
// 指定要拷贝的文件夹路径
File sourceFolder = new File("/path/to/source/folder");
// 指定目标文件夹路径
File destinationFolder = new File("/path/to/destination/folder");
// 如果目标文件夹不存在,则创建它
if (!destinationFolder.exists()) {
destinationFolder.mkdir();
}
// 获取源文件夹下的所有文件和文件夹
File[] files = sourceFolder.listFiles();
for (File file : files) {
// 如果是文件夹,则递归调用本方法
if (file.isDirectory()) {
copyDirectory(file, new File(destinationFolder, file.getName()));
} else {
// 如果是文件,则使用文件流进行拷贝
try (InputStream in = new FileInputStream(file);
OutputStream out = new FileOutputStream(new File(destinationFolder, file.getName()))) {
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
上面的代码中,我们使用了递归的方式来处理文件夹内的所有文件和文件夹。对于文件夹,我们递归调用本方法来处理;对于文件,我们使用文件流进行拷贝。
### 回答2:
要实现拷贝当前工作空间的所有文件(包括目录),可以使用Java的IO流和递归方法来完成。
首先,获取当前工作空间的路径:
```java
String workspacePath = System.getProperty("user.dir");
```
然后,创建一个新的目标文件夹来存放拷贝后的文件:
```java
String targetPath = "拷贝目标文件夹的路径";
File targetFolder = new File(targetPath);
targetFolder.mkdirs();
```
接下来,编写一个递归方法来遍历工作空间的所有文件和文件夹,并进行拷贝:
```java
public void copyFiles(File source, File target) throws IOException {
if (source.isDirectory()) {
if (!target.exists()) {
target.mkdir();
}
String[] files = source.list();
for (String file : files) {
File sourceFile = new File(source, file);
File targetFile = new File(target, file);
copyFiles(sourceFile, targetFile);
}
} else {
InputStream in = new FileInputStream(source);
OutputStream out = new FileOutputStream(target);
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
}
}
```
最后,调用这个方法,传入当前工作空间和目标文件夹的路径,即可完成拷贝:
```java
File sourceFolder = new File(workspacePath);
copyFiles(sourceFolder, targetFolder);
```
以上就是用Java实现拷贝当前工作空间所有文件(含目录)的方法,通过递归遍历工作空间的所有文件和文件夹,并使用IO流进行拷贝。
### 回答3:
要实现拷贝当前工作空间中的所有文件(包括目录),可以使用Java中的File类和IO流进行操作。
首先,需要获取当前工作空间的路径,并创建一个File对象来表示当前路径。可以使用System类中的getProperty()方法来获取系统的工作目录。示例代码如下:
```java
String workspacePath = System.getProperty("user.dir");
File workspace = new File(workspacePath);
```
接下来,可以使用递归的方式遍历当前工作空间的所有文件和目录,将它们拷贝到目标文件夹中。可以使用File类的listFiles()方法获取当前文件夹下的所有子文件和子目录。需要注意的是,拷贝目录时需要先创建目标目录,在拷贝文件时需要创建目标文件。示例代码如下:
```java
public static void copyWorkspaceFiles(File source, File target) throws IOException {
if (source.isDirectory()) {
if (!target.exists()) {
target.mkdir();
}
File[] files = source.listFiles();
for (File file : files) {
File newFile = new File(target.getPath() + File.separator + file.getName());
copyWorkspaceFiles(file, newFile);
}
} else {
InputStream in = new FileInputStream(source);
OutputStream out = new FileOutputStream(target);
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
}
}
// 测试
public static void main(String[] args) {
try {
String workspacePath = System.getProperty("user.dir");
File workspace = new File(workspacePath);
File target = new File("目标文件夹的路径");
copyWorkspaceFiles(workspace, target);
System.out.println("拷贝完成!");
} catch (IOException e) {
e.printStackTrace();
}
}
```
以上代码会将当前工作空间中的所有文件和目录拷贝到指定的目标文件夹中。可以根据实际需求修改代码,比如设置拷贝过滤规则,控制是否拷贝隐藏文件等。
相关推荐
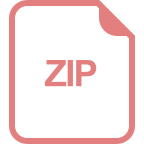
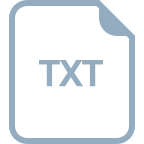
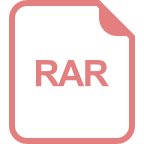














