如何用C++实现线程池
时间: 2023-09-12 18:04:55 浏览: 119
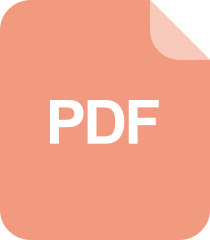
c++实现简单的线程池
### 回答1:
实现线程池的方法有很多,但是最常见的方法是使用队列来维护任务。每个线程都在队列中等待任务,当有新任务到达时,就从队列中取出一个任务并执行。这样,每个线程都可以在并行执行任务,而不需要创建新的线程。
在C语言中实现线程池的代码如下:
```
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#define NUM_THREADS 5
void *print_hello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello World! It's me, thread #%ld!\n", tid);
pthread_exit(NULL);
}
int main(int argc, char *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
printf("In main: creating thread %ld\n", t);
rc = pthread_create(&threads[t], NULL, print_hello, (void *)t);
if (rc) {
printf("ERROR; return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
pthread_exit(NULL);
}
```
### 回答2:
要实现线程池,首先需要先了解线程池的基本概念和原理。
线程池是一种用来管理和复用线程的技术,它能够维护一个线程队列,按需创建和销毁线程,并将任务分配给这些线程来执行。使用线程池可以提高程序的性能,减少线程创建和销毁的开销。
在C语言中,可以使用多线程的库来实现线程池,比如pthread库。下面是一个简单的用C语言实现线程池的步骤:
1. 定义线程池结构体:创建一个结构体来保存线程池的相关信息,如线程池的大小、任务队列、互斥锁、条件变量等。
2. 初始化线程池:在初始化函数中,需要对线程池中的各个成员进行初始化,如创建指定数量的线程、初始化互斥锁和条件变量等。
3. 定义任务函数:线程池的任务函数用于处理任务队列中的任务,根据具体需求来定义任务的执行逻辑。
4. 添加任务到线程池:当有新的任务时,将任务添加到任务队列中,并通过条件变量来通知线程池中的线程有新任务可执行。
5. 线程池中的线程获取任务并执行:在线程中循环检查任务队列,当有任务时,线程从任务队列中获取任务并执行。
6. 销毁线程池:在停止使用线程池时,要销毁线程池中的资源,包括线程的回收、互斥锁和条件变量的销毁等。
通过以上步骤,就可以在C语言中实现一个简单的线程池。具体实现中还需要考虑线程同步、任务队列的管理等问题,以确保线程池的稳定性和性能。
### 回答3:
线程池是用来管理和复用线程的一种机制,可以更有效地使用系统资源和提高应用程序的性能。下面是使用C语言实现线程池的一般步骤:
1. 定义一个线程池的结构体,包含线程池的状态、大小、最大线程数、工作任务队列等信息。
```c
typedef struct {
pthread_t *threads; // 线程数组
int thread_count; // 线程数
int max_threads; // 最大线程数
int pool_size; // 线程池大小
int shutdown; // 关闭标志
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t notify; // 条件变量
Task *task_queue; // 任务队列
} ThreadPool;
```
2. 初始化线程池,创建指定数量的线程。
```c
ThreadPool* thread_pool_init(int pool_size) {
ThreadPool *pool = malloc(sizeof(ThreadPool));
pool->threads = malloc(pool_size * sizeof(pthread_t));
pool->thread_count = pool_size;
pool->max_threads = pool_size;
pool->pool_size = 0;
pool->shutdown = 0;
// 初始化互斥锁和条件变量
pthread_mutex_init(&(pool->mutex), NULL);
pthread_cond_init(&(pool->notify), NULL);
// 创建线程
for (int i = 0; i < pool_size; i++) {
pthread_create(&(pool->threads[i]), NULL, thread_worker, (void*)pool);
}
return pool;
}
```
3. 定义线程工作函数,不断从任务队列中取出任务执行。
```c
void* thread_worker(void *arg) {
ThreadPool *pool = (ThreadPool*)arg;
while (1) {
pthread_mutex_lock(&(pool->mutex));
// 线程池关闭,退出线程
while (pool->pool_size == 0 && !pool->shutdown) {
pthread_cond_wait(&(pool->notify), &(pool->mutex));
}
if (pool->shutdown) {
pthread_mutex_unlock(&(pool->mutex));
pthread_exit(NULL);
}
// 从任务队列中取出任务执行
Task *task = pool->task_queue;
pool->task_queue = pool->task_queue->next;
pool->pool_size--;
pthread_mutex_unlock(&(pool->mutex));
task->func(task->arg);
free(task);
}
pthread_exit(NULL);
}
```
4. 定义任务结构体,包含任务函数指针和参数。
```c
typedef struct Task {
void (*func)(void*);
void *arg;
struct Task *next;
} Task;
```
5. 向线程池中添加任务。
```c
void thread_pool_add_task(ThreadPool *pool, void (*func)(void*), void *arg) {
Task *task = malloc(sizeof(Task));
task->func = func;
task->arg = arg;
task->next = NULL;
pthread_mutex_lock(&(pool->mutex));
if (pool->task_queue == NULL) {
pool->task_queue = task;
} else {
Task *cur = pool->task_queue;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = task;
}
pool->pool_size++;
pthread_mutex_unlock(&(pool->mutex));
pthread_cond_signal(&(pool->notify));
}
```
6. 关闭线程池。
```c
void thread_pool_shutdown(ThreadPool *pool) {
if (pool == NULL) {
return;
}
pthread_mutex_lock(&(pool->mutex));
pool->shutdown = 1;
pthread_mutex_unlock(&(pool->mutex));
pthread_cond_broadcast(&(pool->notify));
// 等待线程退出
for (int i = 0; i < pool->thread_count; i++) {
pthread_join(pool->threads[i], NULL);
}
// 释放资源
free(pool->threads);
while (pool->task_queue != NULL) {
Task *next = pool->task_queue->next;
free(pool->task_queue);
pool->task_queue = next;
}
pthread_mutex_destroy(&(pool->mutex));
pthread_cond_destroy(&(pool->notify));
free(pool);
}
```
以上是一个简单的线程池的实现,通过初始化线程池、添加任务、关闭线程池等操作,可以有效地管理和复用线程,提高应用程序的性能。
阅读全文
相关推荐
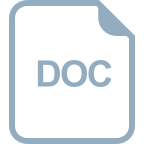
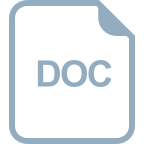


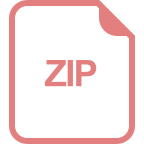
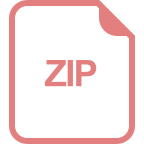
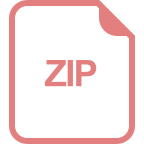
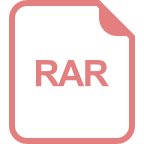
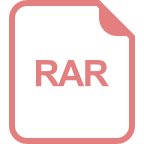
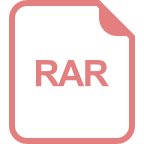
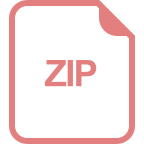
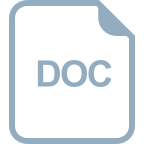

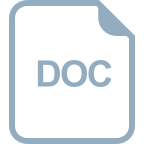