用python写一个ascii转字符串的带界面的程序
时间: 2023-09-03 07:27:38 浏览: 64
### 回答1:
好的,这是一个用 Python 写的 ASCII 转字符串的带界面的程序的示例:
```python
# 导入所需的库
import tkinter as tk
# 创建窗口
window = tk.Tk()
window.title("ASCII 转字符串")
# 创建输入框
ascii_code = tk.StringVar()
ascii_entry = tk.Entry(window, textvariable=ascii_code)
ascii_entry.pack()
# 创建转换按钮
def convert():
# 获取输入的 ASCII 码并转换为字符串
ascii_str = ascii_code.get()
result = "".join(chr(int(c)) for c in ascii_str.split())
# 将转换结果显示在文本框中
result_text.configure(state="normal")
result_text.delete("1.0", "end")
result_text.insert("end", result)
result_text.configure(state="disabled")
convert_button = tk.Button(window, text="转换", command=convert)
convert_button.pack()
# 创建结果文本框
result_text = tk.Text(window)
result_text.configure(state="disabled")
result_text.pack()
# 运行窗口
window.mainloop()
```
这个程序创建了一个包含输入框、转换按钮和结果文本框的窗口。输入框用于输入 ASCII 码,转换按钮用于执行转换,结果文本框用于显示转换结果。转换按钮按下时,程序会将输入的 ASCII 码转换为字符串并显示在结果文本框中。
### 回答2:
要用Python编写一个带界面的ASCII转字符串的程序,你可以使用Python的图形用户界面库如Tkinter或PyQt等。
下面是一个使用Tkinter库实现的示例程序:
```
import tkinter as tk
def ascii_to_string():
ascii_text = ascii_entry.get("1.0", tk.END).strip()
char_text = ""
for value in ascii_text.split():
try:
char_code = int(value)
char_text += chr(char_code)
except ValueError:
pass
string_result.delete("1.0", tk.END)
string_result.insert(tk.END, char_text)
# 创建主窗口
root = tk.Tk()
root.title("ASCII转字符串")
# 创建输入框与按钮及结果展示框
ascii_label = tk.Label(root, text="ASCII序列:")
ascii_label.pack()
ascii_entry = tk.Text(root, height=5)
ascii_entry.pack()
convert_button = tk.Button(root, text="转换", command=ascii_to_string)
convert_button.pack()
result_label = tk.Label(root, text="字符串结果:")
result_label.pack()
string_result = tk.Text(root, height=5)
string_result.pack()
# 进入消息循环
root.mainloop()
```
这个程序创建了一个简单的Tkinter界面,其中用户可以在ASCII序列的输入框中输入一系列的ASCII码,然后点击"转换"按钮,程序会将ASCII码转换为对应的字符,并将结果展示在字符串结果的框中。
你可以运行这个程序,并在ASCII序列的输入框中输入一些数字,点击"转换"按钮即可看到转换后的字符串结果的展示。
希望这个程序能帮到你!
### 回答3:
要用Python编写一个带界面的ASCII转字符串程序,可以利用Python的GUI库来实现。下面是一个示例程序,使用了Tkinter库来创建界面。
```python
import tkinter as tk
def convert_ascii():
ascii_text = ascii_entry.get()
string_text = ""
for code in ascii_text.split():
try:
string_text += chr(int(code))
except:
string_text += "?"
string_entry.delete(0, tk.END)
string_entry.insert(tk.END, string_text)
# 创建主窗口
root = tk.Tk()
root.title("ASCII转字符串")
# 创建ASCII输入框和转换按钮
ascii_label = tk.Label(root, text="ASCII码:")
ascii_label.pack()
ascii_entry = tk.Entry(root)
ascii_entry.pack()
convert_button = tk.Button(root, text="转换", command=convert_ascii)
convert_button.pack()
# 创建字符串文本框
string_label = tk.Label(root, text="字符结果:")
string_label.pack()
string_entry = tk.Entry(root)
string_entry.pack()
# 运行窗口
root.mainloop()
```
这个程序中,我们使用了Tkinter库来创建主窗口、标签、文本框和按钮。用户可以输入ASCII码,并点击转换按钮来将其转换为对应的字符串。转换后的结果将会显示在下方的字符串文本框中。
你可以根据需要自定义界面的布局和样式,这只是一个简单的示例程序。
相关推荐
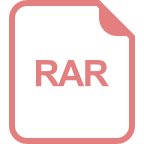
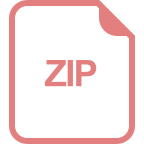
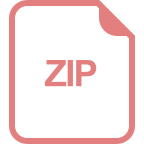














