用python写一个Tkinter库来创建图形化界面,用户可以在界面中输入明文/密文和密钥,然后选择加或解密按钮。加解密算法使用简单的凯撒密码实现。
时间: 2024-04-30 07:20:27 浏览: 9
好的,这里是一个使用 Tkinter 库的示例代码,用于实现凯撒密码的加解密功能:
```python
import tkinter as tk
def encrypt(plaintext, key):
ciphertext = ""
for char in plaintext:
if char.isalpha():
char_code = ord(char.lower()) - 97
new_char_code = (char_code + key) % 26
new_char = chr(new_char_code + 97)
ciphertext += new_char.upper() if char.isupper() else new_char
else:
ciphertext += char
return ciphertext
def decrypt(ciphertext, key):
plaintext = ""
for char in ciphertext:
if char.isalpha():
char_code = ord(char.lower()) - 97
new_char_code = (char_code - key) % 26
new_char = chr(new_char_code + 97)
plaintext += new_char.upper() if char.isupper() else new_char
else:
plaintext += char
return plaintext
def encrypt_button_click():
plaintext = plaintext_entry.get()
key = int(key_entry.get())
ciphertext = encrypt(plaintext, key)
result_label.config(text=ciphertext)
def decrypt_button_click():
ciphertext = plaintext_entry.get()
key = int(key_entry.get())
plaintext = decrypt(ciphertext, key)
result_label.config(text=plaintext)
window = tk.Tk()
window.title("Caesar Cipher")
plaintext_label = tk.Label(window, text="Plaintext/Ciphertext:")
plaintext_label.pack()
plaintext_entry = tk.Entry(window)
plaintext_entry.pack()
key_label = tk.Label(window, text="Key:")
key_label.pack()
key_entry = tk.Entry(window)
key_entry.pack()
encrypt_button = tk.Button(window, text="Encrypt", command=encrypt_button_click)
encrypt_button.pack()
decrypt_button = tk.Button(window, text="Decrypt", command=decrypt_button_click)
decrypt_button.pack()
result_label = tk.Label(window, text="")
result_label.pack()
window.mainloop()
```
代码中使用了 `encrypt` 和 `decrypt` 函数来实现加密和解密操作,这两个函数都接收明文/密文和密钥作为参数,并返回相应的结果。在界面中,我们使用 `tk.Entry` 组件来接收用户输入的明文/密文和密钥,使用 `tk.Button` 组件来触发加解密操作,并使用 `tk.Label` 组件来显示结果。
注意,在代码中使用了 `ord` 和 `chr` 函数来将字符转换为 ASCII 码和反向转换。同时,我们也要注意处理大小写字母的情况,以及非字母字符的情况。
相关推荐
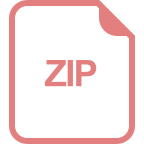
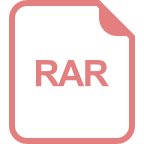
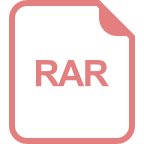














