盒装饮料 自动售货机的JAVA单元测试代码
时间: 2024-09-26 16:00:49 浏览: 37
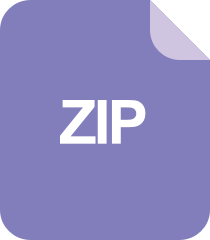
单价为5角的饮料自动售货机

盒装饮料自动售货机的Java单元测试通常会针对关键功能模块编写,例如货币识别、商品选择、付款处理等。下面是一个简化的例子,假设我们有一个`CoinDetector`类负责接收硬币并返回总金额,以及一个`DrinkMachine`类负责售出饮料:
```java
// CoinDetector.java
public class CoinDetector {
public int detectCoins(Coin[] coins) {
// ...实际的硬币检测逻辑...
}
}
// DrinkMachine.java
public class DrinkMachine {
private final CoinDetector detector;
public DrinkMachine(CoinDetector detector) {
this.detector = detector;
}
public void purchase(Drink drink, Coin[] coins) {
int amountDue = drink.getPrice();
int totalCollected = detector.detectCoins(coins);
if (totalCollected >= amountDue) {
dispenseDrink(drink);
} else {
throw new InsufficientFundsException();
}
}
}
// Unit test for DrinkMachine
import org.junit.Test;
import static org.junit.Assert.*;
public class DrinkMachineTest {
@Test
public void testPurchaseSuccess() {
// 测试有足够的硬币购买一瓶水
CoinDetector mockDetector = mock(CoinDetector.class); // 创建模拟对象
when(mockDetector.detectCoins(any())).thenReturn(50); // 模拟检测结果
DrinkMachine machine = new DrinkMachine(mockDetector);
Coin[] coins = {new Quarter(), new Nickel(), new Penny()};
machine.purchase(new Water(), coins); // 饮料应该是成功购买
verify(mockDetector).detectCoins(coins); // 确保检测方法被调用
}
@Test
public void testPurchaseInsufficientFunds() {
// 测试硬币不足的情况
CoinDetector mockDetector = mock(CoinDetector.class);
when(mockDetector.detectCoins(any())).thenReturn(10); // 模拟不足以支付的金额
DrinkMachine machine = new DrinkMachine(mockDetector);
try {
machine.purchase(new Water(), coins); // 预期会抛出异常
fail("Expected an InsufficientFundsException");
} catch (InsufficientFundsException e) {
// 正确的行为
}
}
}
```
阅读全文
相关推荐
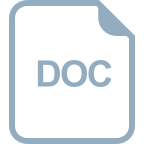
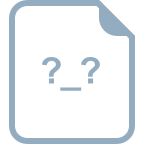



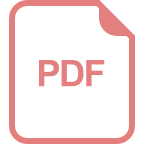
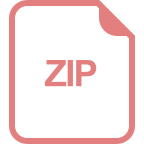
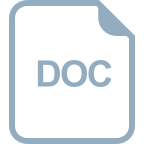
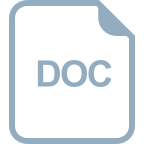






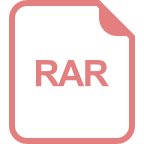