设计一个中英文词典c语言
时间: 2024-11-06 11:19:54 浏览: 14
设计一个中英文词典的C语言程序,通常会包含以下几个步骤:
1. 数据结构选择:可以使用链表、数组结合哈希表的方式来存储数据。每个单词(包括中英文)作为一个节点,包含单词的英文翻译。
```c
typedef struct {
char *word;
char *translation;
} DictionaryEntry;
```
2. 功能模块划分:
- **添加词汇**:用户输入中文,查找对应的英文翻译并添加到字典中。
- **查询翻译**:接收用户输入的英文,查找并返回相应的中文解释。
- **维护字典**:删除、更新单词等功能。
3. 文件操作:如果需要持久化存储,可以考虑将数据保存到文件中,比如JSON或者CSV格式,读取时加载进内存。
4. 用户交互界面:编写命令行交互函数,允许用户输入查询或操作指令。
5. 错误处理:对用户的输入进行有效性检查,并处理可能出现的错误情况。
下面是一个简单的示例,仅展示如何初始化字典和添加元素(实际完整项目需更复杂):
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// 定义字典结构体
typedef struct {
DictionaryEntry *entries; // 链表头部
int count;
} Dictionary;
void addWord(Dictionary *dict, const char *chinese, const char *english) {
DictionaryEntry *new_entry = (DictionaryEntry *)malloc(sizeof(DictionaryEntry));
new_entry->word = strdup(chinese);
new_entry->translation = strdup(english);
if (!dict->entries) {
dict->entries = new_entry;
} else {
DictionaryEntry *last = dict->entries;
while (last->next != NULL) last = last->next;
last->next = new_entry;
}
dict->count++;
}
// ... 其他功能如查询和文件操作
int main() {
Dictionary dict;
dict.entries = NULL;
dict.count = 0;
// 初始化字典,添加一些词汇...
addWord(&dict, "你好", "Hello");
addWord(&dict, "世界", "World");
// 使用字典
// ...
return 0;
}
```
阅读全文
相关推荐
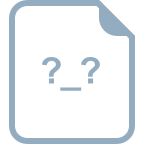
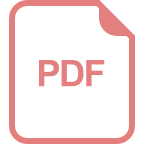
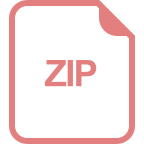
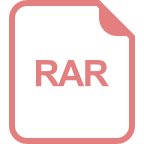
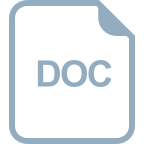
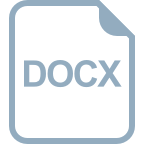
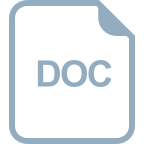
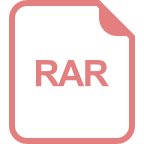
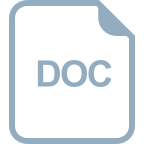
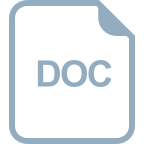
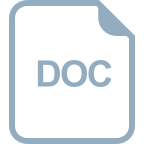
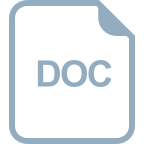
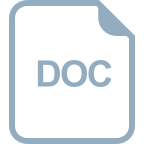
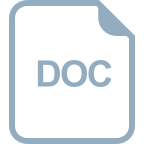
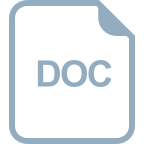
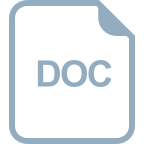
