用循环结构计算斐波那契数列(Fibonacci)的第 n 个值,斐波那契数列满足如下规律, 1,1,2,3,5,8,13,21,34,55,....
时间: 2024-10-09 13:00:56 浏览: 21
斐波那契数列是一个经典的数学序列,每个数字都是前两个数字之和。要使用循环结构计算第 n 个斐波那契数值,你可以用迭代的方法,避免了递归带来的性能开销。以下是一个简单的 Java 代码示例:
```java
public class Fibonacci {
public static int fibonacci(int n) {
if (n <= 0) {
throw new IllegalArgumentException("n must be a positive integer");
} else if (n == 1 || n == 2) {
return n - 1; // Fibonacci sequence starts with 0 and 1, so adjust for the index
}
int prev = 0;
int current = 1;
for (int i = 3; i <= n; i++) {
int temp = current;
current += prev;
prev = temp;
}
return current;
}
public static void main(String[] args) {
int n = 10; // Replace this with the desired value of n
System.out.println("The " + n + "th Fibonacci number is: " + fibonacci(n));
}
}
```
在这个代码中,我们首先检查 n 是否为正整数,然后用一个 `for` 循环从第三个斐波那契数开始计算,每次更新 `prev` 和 `current` 的值,直到得到第 n 个数。
阅读全文
相关推荐
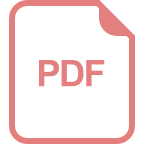
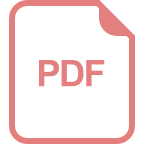
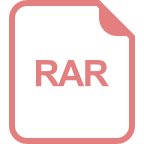















